Calculate Well Trajectory¶
Calculate the well trajectory points from a json document with only wellname, md, inc, azim, and surface latitude and longitude or surface x and y.
Directional surveys come in a variety of formats, these formats are often missing critical information the user needs for their analysis. The welltrajconvert package allows the user to take the bare minimum required wellbore survey information and convert that into its latitude and longitude points along the wellbore and a host of other common parameters.
welltrajconvert requires the wellId, measured depth, inclination angle, azimuth degrees, surface_latitude, and surface_longitude points or surface x and y points to calculate various points along the wellbore using a minimum curvature algorithm.
welltrajconvert calculates the following:
tvd:
true vertical depth from surface to the survey point.
n_s_deviation:
north south deviation for each point in the wellbore path.
e_w_deviation:
east west deviation for each point in the wellbore path.
dls:
Dogleg severity is a measure of the change in direction of a wellbore over a defined length, measured in degrees per 100 feet of length.
surface_x:
Surface Easting component of the UTM coordinate
surface_y:
Surface Northing component of the UTM coordinate
x_points:
Easting component of the UTM coordinate
y_points:
Northing component of the UTM coordinate
zone_number:
Zone number of the UTM coordinate
zone_letter:
Zone letter of the UTM coordinate
latitude_points:
The latitude value of a location in the borehole. A positive value denotes north. Angle subtended with equatorial plane by a perpendicular from a point on the surface of a spheriod.
longitude_points:
The longitude value of a location in a borehole. A positive value denotes east. Angle measured about the spheroid axis from a local prime meridian to the meridian through the point.
isHorizontal:
Array of strings, Vertical Or Horizontal depending on Inclination angle point.
[1]:
from matplotlib import pyplot
from mpl_toolkits.mplot3d import Axes3D
[2]:
from welltrajconvert.wellbore_trajectory import *
Get Wellbore Trajectory object¶
get the path to the json file
[3]:
json_path = './data/wellbore_survey.json'
json_path
[3]:
'./data/wellbore_survey.json'
Open the json, just to take a look at it. the from_json()
takes care of this
[4]:
with open(json_path) as json_file:
json_obj = json.load(json_file)
json_file.close()
# take a look at the json
#print(json.dumps(json_obj, indent=4))
using the WellboreTrajectory class, use from_json
to take the path and convert it to a deviation survey object. This step will validate if the json contains the correct data for the minimum curvature calculation.
Create Wellbore_Survey_Object:
[5]:
# create a wellbore deviation object from the json path
dev_obj = WellboreTrajectory.from_json(json_path)
# take a look at the data
dev_obj.deviation_survey_obj
[5]:
DeviationSurvey(wellId='well_C', md=array([ 0. , 35. , 774.81, 800. , 900. , 1000. ,
1100. , 1200. , 1300. , 1400. , 1500. , 1600. ,
1700. , 1800. , 1900. , 2000. , 2100. , 2200. ,
2300. , 2400. , 2500. , 2600. , 2700. , 2800. ,
2900. , 3000. , 3100. , 3200. , 3300. , 3400. ,
3500. , 3600. , 3700. , 3800. , 3900. , 4000. ,
4100. , 4200. , 4300. , 4400. , 4500. , 4600. ,
4700. , 4800. , 4900. , 5000. , 5100. , 5200. ,
5300. , 5400. , 5500. , 5600. , 5700. , 5800. ,
5900. , 6000. , 6100. , 6200. , 6300. , 6400. ,
6450.67, 6532. , 6625. , 6720. , 6813. , 6909. ,
7002. , 7098. , 7191. , 7286. , 7379. , 7475. ,
7569. , 7663. , 7758. , 7851. , 7947. , 8040. ,
8135. , 8229. , 8324. , 8418. , 8512. , 8606. ,
8700. , 8794. , 8859. , 8924. , 9018. , 9112. ,
9206. , 9292. , 9386. , 9481. , 9574. , 9669. ,
9764. , 9858. , 9952. , 10047. , 10142. , 10236. ,
10330. , 10424. , 10517. , 10613. , 10707. , 10802. ,
10851. , 10890. ]), inc=array([ 0. , 0. , 0.46, 0.13, 0.57, 0.49, 0.29, 0.6 , 0.53,
0.83, 0.61, 0.87, 1.17, 1.27, 1.21, 1.18, 1.24, 1.07,
1.14, 1.16, 0.86, 0.95, 1.22, 0.82, 0.83, 0.98, 0.87,
1.25, 1.06, 1.07, 1.34, 1.03, 1.17, 1.34, 1.37, 1.39,
1.16, 1.61, 1.58, 1.78, 1.78, 1.81, 1.86, 1.63, 1.51,
1.57, 1.09, 0.99, 0.88, 0.91, 0.83, 0.77, 0.64, 0.91,
0.94, 0.66, 1.09, 0.91, 0.95, 0.8 , 4.91, 10.35, 15.28,
20.67, 26.19, 34.23, 40.44, 44.43, 45.39, 48.78, 51.19, 53.89,
56.1 , 58.69, 61.62, 64.38, 67.3 , 70.15, 72.39, 75.47, 78.67,
81.46, 84.59, 86.2 , 87.65, 89.13, 90.27, 89.72, 89.9 , 90.02,
90.05, 90.03, 89.94, 90.06, 90.37, 90.24, 90.06, 89.97, 89.88,
90.15, 90.03, 89.91, 89.97, 90. , 89.88, 89.88, 90. , 89.72,
90.06, 90. ]), azim=array([227.11, 227.11, 227.11, 163.86, 230.43, 254.47, 268.25, 147.67,
236.56, 190.05, 342.93, 184.69, 187.06, 193.66, 195.77, 185.77,
214.81, 203.82, 189.81, 194.18, 109.65, 214.46, 205.35, 190.63,
187.75, 193.45, 188.19, 205.4 , 130.09, 218.09, 247.16, 245.17,
239.46, 234.52, 232.45, 228.1 , 199.28, 305.98, 213.22, 211.63,
207.08, 209.84, 204.39, 208. , 208.46, 201.98, 187.95, 185.23,
165.95, 163.86, 138.75, 253.13, 132.16, 189.07, 141.79, 143.34,
306.53, 111.26, 117.69, 87.41, 55.44, 48.23, 50.93, 49.37,
48.77, 48.96, 48.41, 48.88, 49.58, 49.67, 49.73, 49.78,
49.14, 49.25, 49.5 , 49.12, 48.63, 48.79, 48.83, 49.02,
49.49, 49.55, 49.29, 47.62, 47.22, 47.69, 48.36, 48.41,
44.97, 42.69, 40.86, 40.48, 40.36, 39.41, 38.74, 40.09,
38.63, 35.85, 33.71, 32.12, 30.91, 29.75, 28.78, 28.53,
29.28, 27.59, 26.69, 26.72, 27.04, 27.35]), surface_latitude=29.90829444, surface_longitude=47.68852083, tvd=None, n_s_deviation=None, e_w_deviation=None, dls=None, surface_x=None, surface_y=None, x_points=None, y_points=None, zone_number=None, zone_letter=None, latitude_points=None, longitude_points=None, isHorizontal=None)
As you can see above, there is only input data for wellId, md, inc, azim, and surface latitude and longitude. The rest of the data is missing and needs to be calculated.
Calculate Survey Points:
[6]:
# now you can calculate the survey points using a minimum curvature algorithm
dev_obj.calculate_survey_points()
# take a look at the data that was just calculated
dev_obj.deviation_survey_obj
[6]:
DeviationSurvey(wellId='well_C', md=array([ 0. , 35. , 774.81, 800. , 900. , 1000. ,
1100. , 1200. , 1300. , 1400. , 1500. , 1600. ,
1700. , 1800. , 1900. , 2000. , 2100. , 2200. ,
2300. , 2400. , 2500. , 2600. , 2700. , 2800. ,
2900. , 3000. , 3100. , 3200. , 3300. , 3400. ,
3500. , 3600. , 3700. , 3800. , 3900. , 4000. ,
4100. , 4200. , 4300. , 4400. , 4500. , 4600. ,
4700. , 4800. , 4900. , 5000. , 5100. , 5200. ,
5300. , 5400. , 5500. , 5600. , 5700. , 5800. ,
5900. , 6000. , 6100. , 6200. , 6300. , 6400. ,
6450.67, 6532. , 6625. , 6720. , 6813. , 6909. ,
7002. , 7098. , 7191. , 7286. , 7379. , 7475. ,
7569. , 7663. , 7758. , 7851. , 7947. , 8040. ,
8135. , 8229. , 8324. , 8418. , 8512. , 8606. ,
8700. , 8794. , 8859. , 8924. , 9018. , 9112. ,
9206. , 9292. , 9386. , 9481. , 9574. , 9669. ,
9764. , 9858. , 9952. , 10047. , 10142. , 10236. ,
10330. , 10424. , 10517. , 10613. , 10707. , 10802. ,
10851. , 10890. ]), inc=array([ 0. , 0. , 0.46, 0.13, 0.57, 0.49, 0.29, 0.6 , 0.53,
0.83, 0.61, 0.87, 1.17, 1.27, 1.21, 1.18, 1.24, 1.07,
1.14, 1.16, 0.86, 0.95, 1.22, 0.82, 0.83, 0.98, 0.87,
1.25, 1.06, 1.07, 1.34, 1.03, 1.17, 1.34, 1.37, 1.39,
1.16, 1.61, 1.58, 1.78, 1.78, 1.81, 1.86, 1.63, 1.51,
1.57, 1.09, 0.99, 0.88, 0.91, 0.83, 0.77, 0.64, 0.91,
0.94, 0.66, 1.09, 0.91, 0.95, 0.8 , 4.91, 10.35, 15.28,
20.67, 26.19, 34.23, 40.44, 44.43, 45.39, 48.78, 51.19, 53.89,
56.1 , 58.69, 61.62, 64.38, 67.3 , 70.15, 72.39, 75.47, 78.67,
81.46, 84.59, 86.2 , 87.65, 89.13, 90.27, 89.72, 89.9 , 90.02,
90.05, 90.03, 89.94, 90.06, 90.37, 90.24, 90.06, 89.97, 89.88,
90.15, 90.03, 89.91, 89.97, 90. , 89.88, 89.88, 90. , 89.72,
90.06, 90. ]), azim=array([227.11, 227.11, 227.11, 163.86, 230.43, 254.47, 268.25, 147.67,
236.56, 190.05, 342.93, 184.69, 187.06, 193.66, 195.77, 185.77,
214.81, 203.82, 189.81, 194.18, 109.65, 214.46, 205.35, 190.63,
187.75, 193.45, 188.19, 205.4 , 130.09, 218.09, 247.16, 245.17,
239.46, 234.52, 232.45, 228.1 , 199.28, 305.98, 213.22, 211.63,
207.08, 209.84, 204.39, 208. , 208.46, 201.98, 187.95, 185.23,
165.95, 163.86, 138.75, 253.13, 132.16, 189.07, 141.79, 143.34,
306.53, 111.26, 117.69, 87.41, 55.44, 48.23, 50.93, 49.37,
48.77, 48.96, 48.41, 48.88, 49.58, 49.67, 49.73, 49.78,
49.14, 49.25, 49.5 , 49.12, 48.63, 48.79, 48.83, 49.02,
49.49, 49.55, 49.29, 47.62, 47.22, 47.69, 48.36, 48.41,
44.97, 42.69, 40.86, 40.48, 40.36, 39.41, 38.74, 40.09,
38.63, 35.85, 33.71, 32.12, 30.91, 29.75, 28.78, 28.53,
29.28, 27.59, 26.69, 26.72, 27.04, 27.35]), surface_latitude=29.90829444, surface_longitude=47.68852083, tvd=array([ 0. , 35. , 774.80205236, 799.9916839 ,
899.98957066, 999.98528439, 1099.98291708, 1199.97977187,
1299.97490562, 1399.96774509, 1499.95980744, 1599.95135856,
1699.93539853, 1799.91271696, 1899.88929431, 1999.86754393,
2099.8452397 , 2199.8248857 , 2299.80628255, 2399.78613986,
2499.77051364, 2599.75802178, 2699.73999928, 2799.7239516 ,
2899.71358513, 2999.70108231, 3099.68803535, 3199.67073753,
3299.65039143, 3399.63311651, 3499.6109049 , 3599.58939615,
3699.5709424 , 3799.54691757, 3899.51895345, 3999.48994863,
4099.46512692, 4199.43556378, 4299.39682997, 4399.35379463,
4499.30554098, 4599.25646956, 4699.20518489, 4799.15874306,
4899.12118453, 4999.0850605 , 5099.05782961, 5199.04134366,
5299.02801386, 5399.01581265, 5499.004277 , 5598.99453025,
5698.9869478 , 5798.9777011 , 5898.96466764, 5998.95482054,
6098.94287286, 6198.92763525, 6298.9144603 , 6398.90277221,
6449.49892068, 6530.07840696, 6620.73385719, 6711.06365715,
6796.36247083, 6879.25635883, 6953.16472562, 7024.00253299,
7089.86588017, 7154.54311968, 7214.33658158, 7272.71906108,
7326.6385766 , 7377.28559394, 7424.55766084, 7466.7746503 ,
7506.06181579, 7539.80284576, 7570.306201 , 7596.32330294,
7617.57772512, 7633.79396604, 7645.20749536, 7652.75404791,
7657.79630452, 7660.43720229, 7660.77749063, 7660.78311918,
7661.09477093, 7661.16033202, 7661.10284739, 7661.04275014,
7661.06729601, 7661.06723206, 7660.71819198, 7660.2124211 ,
7659.96364797, 7659.93897552, 7660.06195799, 7660.03702311,
7659.88773352, 7659.93688854, 7660.03526184, 7660.05980771,
7660.15713449, 7660.35813173, 7660.45650502, 7660.6885695 ,
7660.7826096 , 7660.76216298]), n_s_deviation=array([ 0.00000000e+00, -1.37853123e-17, -2.02120304e+00, -2.11747423e+00,
-2.54331148e+00, -2.97465803e+00, -3.09687233e+00, -3.54702524e+00,
-4.24431864e+00, -5.21236307e+00, -5.41667042e+00, -5.66445225e+00,
-7.43430965e+00, -9.52436356e+00, -1.16173152e+01, -1.36578743e+01,
-1.55707206e+01, -1.73132762e+01, -1.91476665e+01, -2.11092738e+01,
-2.23430190e+01, -2.32789031e+01, -2.49244904e+01, -2.65898398e+01,
-2.80107915e+01, -2.95601783e+01, -3.11433404e+01, -3.28801005e+01,
-3.44610833e+01, -3.57916156e+01, -3.69803351e+01, -3.78116235e+01,
-3.87078339e+01, -3.99052784e+01, -4.13125015e+01, -4.28510661e+01,
-4.46165238e+01, -4.47466470e+01, -4.50746457e+01, -4.75503611e+01,
-5.02555752e+01, -5.30082836e+01, -5.58562012e+01, -5.85900167e+01,
-6.10041327e+01, -6.34328142e+01, -6.56451723e+01, -6.74474774e+01,
-6.90527190e+01, -7.05604547e+01, -7.18677961e+01, -7.26073384e+01,
-7.31771963e+01, -7.43362244e+01, -7.57649125e+01, -7.68714604e+01,
-7.67673139e+01, -7.64890862e+01, -7.71622488e+01, -7.75159265e+01,
-7.62693198e+01, -6.94230110e+01, -5.61261178e+01, -3.73044917e+01,
-1.30713494e+01, 1.86716088e+01, 5.59039697e+01, 9.86865303e+01,
1.41558635e+02, 1.86620485e+02, 2.32685134e+02, 2.81911427e+02,
3.31957433e+02, 3.83699227e+02, 4.37342727e+02, 4.91364006e+02,
5.48969383e+02, 6.06148249e+02, 6.65394128e+02, 7.24734956e+02,
7.85158398e+02, 8.45260340e+02, 9.05948465e+02, 9.68081147e+02,
1.03158981e+03, 1.09512217e+03, 1.13859248e+03, 1.18176022e+03,
1.24620960e+03, 1.31400750e+03, 1.38410060e+03, 1.44932911e+03,
1.52089238e+03, 1.59378648e+03, 1.66598252e+03, 1.73937026e+03,
1.81281578e+03, 1.88762789e+03, 1.96482110e+03, 2.04456368e+03,
2.12554687e+03, 2.20667702e+03, 2.28867662e+03, 2.37116358e+03,
2.45257604e+03, 2.53698503e+03, 2.62063246e+03, 2.70549867e+03,
2.74920416e+03, 2.78389282e+03]), e_w_deviation=array([ 0.00000000e+00, -1.48400066e-17, -2.17584236e+00, -2.24198402e+00,
-2.59387761e+00, -3.38929521e+00, -4.05423896e+00, -4.02718020e+00,
-4.13311241e+00, -4.64545267e+00, -4.92810207e+00, -5.14643276e+00,
-5.33399368e+00, -5.72119085e+00, -6.26985886e+00, -6.66033428e+00,
-7.38153427e+00, -8.37630369e+00, -8.92288458e+00, -9.34034125e+00,
-8.88154478e+00, -8.64385623e+00, -9.56872314e+00, -1.01565171e+01,
-1.03861865e+01, -1.06827693e+01, -1.09898326e+01, -1.15658473e+01,
-1.13260729e+01, -1.11944362e+01, -1.28480229e+01, -1.47413241e+01,
-1.64363521e+01, -1.82678247e+01, -2.01677492e+01, -2.20182850e+01,
-2.32552747e+01, -2.47263008e+01, -2.66183940e+01, -2.81881843e+01,
-2.97097016e+01, -3.12025354e+01, -3.26585056e+01, -3.39963761e+01,
-3.52919696e+01, -3.64325906e+01, -3.70768846e+01, -3.72871869e+01,
-3.71795109e+01, -3.67723413e+01, -3.60740414e+01, -3.62395027e+01,
-3.64685225e+01, -3.61797082e+01, -3.57975182e+01, -3.49462669e+01,
-3.53666805e+01, -3.53909200e+01, -3.39168180e+01, -3.24853682e+01,
-3.03452994e+01, -2.20237849e+01, -6.26921560e+00, 1.61908433e+01,
4.41039232e+01, 8.04614299e+01, 1.22790651e+02, 1.71410194e+02,
2.21135813e+02, 2.74132499e+02, 3.28451128e+02, 3.86610837e+02,
4.45115521e+02, 5.05050118e+02, 5.67585921e+02, 6.30408504e+02,
6.96378191e+02, 7.61488266e+02, 8.29188492e+02, 8.97273922e+02,
9.67414937e+02, 1.03783540e+03, 1.10869046e+03, 1.17880536e+03,
1.24791863e+03, 1.31714307e+03, 1.36546419e+03, 1.41405959e+03,
1.48242772e+03, 1.54751178e+03, 1.61012728e+03, 1.66617329e+03,
1.72712153e+03, 1.78803807e+03, 1.84665796e+03, 1.90697156e+03,
1.96721460e+03, 2.02408247e+03, 2.07769323e+03, 2.12931067e+03,
2.17896642e+03, 2.22643208e+03, 2.27238229e+03, 2.31745844e+03,
2.36240983e+03, 2.40811628e+03, 2.45099457e+03, 2.49368712e+03,
2.51584102e+03, 2.53366475e+03]), dls=array([0.00000000e+00, 0.00000000e+00, 6.21781757e-02, 1.31232310e+00,
4.39221317e-01, 8.04228773e-02, 2.00071540e-01, 3.05418485e-01,
7.54425181e-02, 2.97606656e-01, 2.36700951e-01, 2.42136276e-01,
2.99985041e-01, 9.98282346e-02, 6.00182291e-02, 3.03785574e-02,
5.67899502e-02, 1.70424766e-01, 6.93668432e-02, 1.99329254e-02,
3.15750269e-01, 7.20972968e-02, 2.69745086e-01, 4.00573314e-01,
9.98500555e-03, 1.49929930e-01, 1.10062745e-01, 3.79150580e-01,
2.07259038e-01, 9.10249869e-03, 2.66848255e-01, 3.10014770e-01,
1.39895762e-01, 1.69898507e-01, 2.99791190e-02, 1.99042910e-02,
2.33485281e-01, 4.08046160e-01, 7.65236333e-02, 1.99981263e-01,
1.74220101e-04, 2.99348167e-02, 4.97345106e-02, 2.30105145e-01,
1.20001478e-01, 5.97357686e-02, 4.80891203e-01, 1.00021295e-01,
1.10852783e-01, 2.99907267e-02, 8.12457823e-02, 7.57578344e-02,
1.43026417e-01, 2.65385225e-01, 2.51993303e-02, 2.80004182e-01,
4.05427058e-01, 2.14009347e-01, 3.99051249e-02, 1.51809635e-01,
8.09081900e+00, 6.68023804e+00, 5.29784122e+00, 5.67160924e+00,
5.93496227e+00, 8.37492511e+00, 6.67638890e+00, 4.15534146e+00,
1.02996749e+00, 3.56838401e+00, 2.59138009e+00, 2.81248790e+00,
2.34851585e+00, 2.75524166e+00, 3.08378139e+00, 2.96666935e+00,
3.03985353e+00, 3.06431010e+00, 2.35788341e+00, 3.27628910e+00,
3.36649771e+00, 2.96805503e+00, 3.32917200e+00, 1.68704987e+00,
1.54107353e+00, 1.57242053e+00, 1.74782160e+00, 8.46188075e-01,
8.16646613e-02, 7.94058502e-02, 8.27467516e-04, 2.47210927e-02,
9.58784409e-02, 1.18025764e-01, 3.29121479e-01, 1.53582345e-01,
2.09053372e-01, 1.67478606e-01, 1.38255244e-01, 2.60989359e-01,
1.39764497e-01, 1.40151347e-01, 5.50950458e-02, 3.13346899e-02,
1.34310492e-01, 2.59607171e-02, 1.20140067e-01, 2.94745341e-01,
6.92054431e-01, 1.55996606e-01]), surface_x=759587.9344401711, surface_y=3311661.864849136, x_points=array([759587.93444017, 759587.93444017, 759587.27124342, 759587.25108344,
759587.14382628, 759586.90138299, 759586.69870814, 759586.70695564,
759586.67466751, 759586.5185062 , 759586.43235466, 759586.36580747,
759586.3086389 , 759586.1906212 , 759586.02338719, 759585.90437028,
759585.68454853, 759585.38134281, 759585.21474495, 759585.08750416,
759585.22734532, 759585.29979279, 759585.01789336, 759584.83873376,
759584.76873053, 759584.67833208, 759584.58473918, 759584.40916992,
759584.48225316, 759584.52237603, 759584.01836278, 759583.44128459,
759582.92464004, 759582.36640719, 759581.78731022, 759581.22326689,
759580.84623245, 759580.39786367, 759579.82115369, 759579.34268161,
759578.87892312, 759578.42390738, 759577.98012767, 759577.57234474,
759577.17744785, 759576.82978656, 759576.63340573, 759576.56930561,
759576.60212525, 759576.72623055, 759576.93907234, 759576.88863974,
759576.8188345 , 759576.9068651 , 759577.02335663, 759577.28281802,
759577.15467595, 759577.14728775, 759577.59659405, 759578.03289993,
759578.68519291, 759581.22159055, 759586.02358326, 759592.86940922,
759601.37731597, 759612.45908399, 759625.3610306 , 759640.18026739,
759655.33663587, 759671.49002588, 759688.04634402, 759705.77342333,
759723.60565099, 759741.87371618, 759760.93462884, 759780.08295207,
759800.19051272, 759820.03606369, 759840.67109264, 759861.4235316 ,
759882.802513 , 759904.2666706 , 759925.86329204, 759947.23431319,
759968.30003949, 759989.39964669, 760004.12792385, 760018.93980192,
760039.77840835, 760059.61603204, 760078.70123438, 760095.78405957,
760114.36108149, 760132.92844506, 760150.79578717, 760169.17937103,
760187.54145013, 760204.87477767, 760221.21533751, 760236.94833312,
760252.08340599, 760266.5509392 , 760280.55656304, 760294.29577225,
760307.99695571, 760321.92828112, 760334.99758426, 760348.01027549,
760354.7627828 , 760360.19545591]), y_points=array([3311661.86484914, 3311661.86484914, 3311661.24878645,
3311661.21944299, 3311661.0896478 , 3311660.95817337,
3311660.92092245, 3311660.78371584, 3311660.57118081,
3311660.27612087, 3311660.21384799, 3311660.13832409,
3311659.59887155, 3311658.96182312, 3311658.32389146,
3311657.70192905, 3311657.11889351, 3311656.58776254,
3311656.02864039, 3311655.4307425 , 3311655.05469696,
3311654.76943947, 3311654.26786448, 3311653.76026595,
3311653.32715989, 3311652.85490678, 3311652.37235899,
3311651.8429945 , 3311651.36111094, 3311650.9555647 ,
3311650.593243 , 3311650.3398663 , 3311650.06670136,
3311649.70172028, 3311649.27279868, 3311648.8038442 ,
3311648.26573267, 3311648.22607114, 3311648.12609713,
3311647.37149908, 3311646.54694983, 3311645.70792429,
3311644.83987901, 3311644.00661204, 3311643.27078948,
3311642.53052736, 3311641.85620062, 3311641.30685803,
3311640.81758037, 3311640.35802254, 3311639.95954488,
3311639.73413239, 3311639.56043972, 3311639.20716795,
3311638.7717038 , 3311638.43442801, 3311638.46617186,
3311638.55097566, 3311638.34579571, 3311638.23799473,
3311638.61796045, 3311640.70471538, 3311644.75760844,
3311650.49444008, 3311657.88070182, 3311667.55595549,
3311678.90437911, 3311691.94450356, 3311705.01192119,
3311718.74677303, 3311732.78727812, 3311747.79145201,
3311763.04547471, 3311778.81637349, 3311795.16691218,
3311811.63259822, 3311829.19071712, 3311846.6188354 ,
3311864.67697941, 3311882.76406374, 3311901.18112871,
3311919.50020064, 3311937.99794123, 3311956.9359827 ,
3311976.29342274, 3311995.658087 , 3312008.90783771,
3312022.06536487, 3312041.7095345 , 3312062.37433424,
3312083.73871144, 3312103.62036305, 3312125.43284558,
3312147.65096739, 3312169.65632205, 3312192.02490452,
3312214.41110004, 3312237.21382894, 3312260.74232019,
3312285.04786023, 3312309.73153467, 3312334.46000463,
3312359.45348241, 3312384.59550716, 3312409.41002751,
3312435.137885 , 3312460.63362334, 3312486.5008434 ,
3312499.82227807, 3312510.39538081]), zone_number=38, zone_letter='R', latitude_points=array([29.90829449, 29.90829449, 29.90828908, 29.90828882, 29.90828767,
29.90828654, 29.90828625, 29.90828501, 29.9082831 , 29.90828047,
29.90827993, 29.90827926, 29.90827441, 29.90826869, 29.90826298,
29.90825739, 29.90825219, 29.90824746, 29.90824246, 29.90823709,
29.90823367, 29.90823109, 29.90822662, 29.90822209, 29.9082182 ,
29.90821396, 29.90820963, 29.90820489, 29.90820053, 29.90819687,
29.90819371, 29.90819155, 29.90818919, 29.90818602, 29.90818228,
29.90817817, 29.9081734 , 29.90817313, 29.90817235, 29.90816565,
29.90815832, 29.90815085, 29.90814312, 29.90813569, 29.90812914,
29.90812254, 29.90811651, 29.90811157, 29.90810715, 29.90810298,
29.90809934, 29.90809732, 29.90809577, 29.90809257, 29.90808862,
29.90808552, 29.90808584, 29.9080866 , 29.90808466, 29.90808359,
29.90808688, 29.90810516, 29.90814068, 29.90819095, 29.90825574,
29.90834062, 29.9084402 , 29.90855463, 29.90866923, 29.90878963,
29.90891271, 29.90904422, 29.90917797, 29.90931628, 29.90945965,
29.90960404, 29.90975808, 29.90991099, 29.91006942, 29.91022809,
29.91038959, 29.9105502 , 29.91071239, 29.91087859, 29.91104864,
29.91121875, 29.91133508, 29.91145056, 29.91162324, 29.91180533,
29.91199389, 29.9121695 , 29.9123622 , 29.91255857, 29.91275316,
29.91295092, 29.91314884, 29.91335073, 29.91355937, 29.91377515,
29.91399446, 29.91421432, 29.91443666, 29.9146604 , 29.9148812 ,
29.91511018, 29.91533725, 29.91556768, 29.91568634, 29.9157805 ]), longitude_points=array([47.68852083, 47.68852083, 47.68851382, 47.6885136 , 47.68851246,
47.68850992, 47.68850782, 47.68850787, 47.68850748, 47.6885058 ,
47.68850489, 47.68850418, 47.68850346, 47.68850208, 47.6885002 ,
47.68849882, 47.6884964 , 47.68849313, 47.68849128, 47.68848981,
47.68849117, 47.68849185, 47.68848881, 47.68848684, 47.68848601,
47.68848496, 47.68848387, 47.68848193, 47.68848257, 47.68848288,
47.68847758, 47.68847155, 47.68846613, 47.68846027, 47.68845417,
47.68844822, 47.68844419, 47.68843954, 47.68843355, 47.68842842,
47.68842342, 47.68841851, 47.68841371, 47.68840928, 47.68840502,
47.68840124, 47.68839905, 47.68839825, 47.68839847, 47.68839965,
47.68840175, 47.68840117, 47.68840041, 47.68840124, 47.68840234,
47.68840494, 47.68840362, 47.68840356, 47.68840816, 47.68841265,
47.68841949, 47.68844624, 47.68849691, 47.68856914, 47.68865896,
47.68877598, 47.68891223, 47.68906873, 47.68922872, 47.6893992 ,
47.68957391, 47.68976098, 47.6899492 , 47.69014205, 47.69034324,
47.69054537, 47.69075769, 47.69096727, 47.69118517, 47.69140429,
47.69162998, 47.69185653, 47.69208449, 47.69231022, 47.6925329 ,
47.69275593, 47.69291155, 47.69306801, 47.69328841, 47.6934987 ,
47.69370137, 47.69388296, 47.69408049, 47.69427802, 47.69446825,
47.69466391, 47.69485935, 47.69504425, 47.69521906, 47.69538777,
47.69555038, 47.6957061 , 47.6958571 , 47.69600538, 47.69615319,
47.6963036 , 47.69644504, 47.69658598, 47.6966591 , 47.69671789]), isHorizontal=array(['Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal'], dtype='<U10'))
As you can see above, the data for latitude, longitude points and various other points along the wellbore have been calculated.
Serialize Dict:
[7]:
# finally, you can serialize the data object back to a json string to export or be used in a other applications.
json_ds = dev_obj.serialize()
json_ds
[7]:
'{"wellId": "well_C", "md": [0.0, 35.0, 774.81, 800.0, 900.0, 1000.0, 1100.0, 1200.0, 1300.0, 1400.0, 1500.0, 1600.0, 1700.0, 1800.0, 1900.0, 2000.0, 2100.0, 2200.0, 2300.0, 2400.0, 2500.0, 2600.0, 2700.0, 2800.0, 2900.0, 3000.0, 3100.0, 3200.0, 3300.0, 3400.0, 3500.0, 3600.0, 3700.0, 3800.0, 3900.0, 4000.0, 4100.0, 4200.0, 4300.0, 4400.0, 4500.0, 4600.0, 4700.0, 4800.0, 4900.0, 5000.0, 5100.0, 5200.0, 5300.0, 5400.0, 5500.0, 5600.0, 5700.0, 5800.0, 5900.0, 6000.0, 6100.0, 6200.0, 6300.0, 6400.0, 6450.67, 6532.0, 6625.0, 6720.0, 6813.0, 6909.0, 7002.0, 7098.0, 7191.0, 7286.0, 7379.0, 7475.0, 7569.0, 7663.0, 7758.0, 7851.0, 7947.0, 8040.0, 8135.0, 8229.0, 8324.0, 8418.0, 8512.0, 8606.0, 8700.0, 8794.0, 8859.0, 8924.0, 9018.0, 9112.0, 9206.0, 9292.0, 9386.0, 9481.0, 9574.0, 9669.0, 9764.0, 9858.0, 9952.0, 10047.0, 10142.0, 10236.0, 10330.0, 10424.0, 10517.0, 10613.0, 10707.0, 10802.0, 10851.0, 10890.0], "inc": [0.0, 0.0, 0.46, 0.13, 0.57, 0.49, 0.29, 0.6, 0.53, 0.83, 0.61, 0.87, 1.17, 1.27, 1.21, 1.18, 1.24, 1.07, 1.14, 1.16, 0.86, 0.95, 1.22, 0.82, 0.83, 0.98, 0.87, 1.25, 1.06, 1.07, 1.34, 1.03, 1.17, 1.34, 1.37, 1.39, 1.16, 1.61, 1.58, 1.78, 1.78, 1.81, 1.86, 1.63, 1.51, 1.57, 1.09, 0.99, 0.88, 0.91, 0.83, 0.77, 0.64, 0.91, 0.94, 0.66, 1.09, 0.91, 0.95, 0.8, 4.91, 10.35, 15.28, 20.67, 26.19, 34.23, 40.44, 44.43, 45.39, 48.78, 51.19, 53.89, 56.1, 58.69, 61.62, 64.38, 67.3, 70.15, 72.39, 75.47, 78.67, 81.46, 84.59, 86.2, 87.65, 89.13, 90.27, 89.72, 89.9, 90.02, 90.05, 90.03, 89.94, 90.06, 90.37, 90.24, 90.06, 89.97, 89.88, 90.15, 90.03, 89.91, 89.97, 90.0, 89.88, 89.88, 90.0, 89.72, 90.06, 90.0], "azim": [227.11, 227.11, 227.11, 163.86, 230.43, 254.47, 268.25, 147.67, 236.56, 190.05, 342.93, 184.69, 187.06, 193.66, 195.77, 185.77, 214.81, 203.82, 189.81, 194.18, 109.65, 214.46, 205.35, 190.63, 187.75, 193.45, 188.19, 205.4, 130.09, 218.09, 247.16, 245.17, 239.46, 234.52, 232.45, 228.1, 199.28, 305.98, 213.22, 211.63, 207.08, 209.84, 204.39, 208.0, 208.46, 201.98, 187.95, 185.23, 165.95, 163.86, 138.75, 253.13, 132.16, 189.07, 141.79, 143.34, 306.53, 111.26, 117.69, 87.41, 55.44, 48.23, 50.93, 49.37, 48.77, 48.96, 48.41, 48.88, 49.58, 49.67, 49.73, 49.78, 49.14, 49.25, 49.5, 49.12, 48.63, 48.79, 48.83, 49.02, 49.49, 49.55, 49.29, 47.62, 47.22, 47.69, 48.36, 48.41, 44.97, 42.69, 40.86, 40.48, 40.36, 39.41, 38.74, 40.09, 38.63, 35.85, 33.71, 32.12, 30.91, 29.75, 28.78, 28.53, 29.28, 27.59, 26.69, 26.72, 27.04, 27.35], "tvd": [0.0, 34.9999999999999, 774.8020523575979, 799.9916838982401, 899.9895706619063, 999.9852843864701, 1099.9829170820146, 1199.9797718735474, 1299.9749056242865, 1399.9677450917852, 1499.9598074391574, 1599.951358560927, 1699.9353985310372, 1799.9127169630583, 1899.889294307163, 1999.8675439283443, 2099.845239700057, 2199.824885701308, 2299.806282545446, 2399.786139863118, 2499.770513641399, 2599.7580217806603, 2699.7399992785035, 2799.7239516033837, 2899.7135851343646, 2999.701082311137, 3099.6880353482165, 3199.6707375309666, 3299.6503914295877, 3399.6331165071, 3499.610904896245, 3599.5893961454594, 3699.5709423951766, 3799.5469175712324, 3899.5189534454853, 3999.489948634858, 4099.465126923902, 4199.4355637827375, 4299.396829968807, 4399.35379463453, 4499.305540982791, 4599.256469555635, 4699.205184887019, 4799.158743060025, 4899.121184533607, 4999.085060495655, 5099.05782961302, 5199.041343661597, 5299.028013859704, 5399.015812645296, 5499.004276998297, 5598.994530251943, 5698.98694779626, 5798.9777010953785, 5898.964667638501, 5998.9548205379915, 6098.942872857037, 6198.927635252819, 6298.914460300661, 6398.9027722133005, 6449.498920678799, 6530.078406955509, 6620.7338571887985, 6711.063657145683, 6796.362470827802, 6879.256358828799, 6953.164725618479, 7024.002532987769, 7089.865880174081, 7154.543119676297, 7214.336581582896, 7272.719061079499, 7326.638576597015, 7377.28559393612, 7424.557660836965, 7466.774650295593, 7506.061815793666, 7539.80284575592, 7570.306200996387, 7596.323302940914, 7617.577725120327, 7633.793966038078, 7645.207495361553, 7652.754047905717, 7657.7963045183415, 7660.437202287923, 7660.7774906349405, 7660.783119177495, 7661.09477092539, 7661.160332020118, 7661.102847387391, 7661.042750144869, 7661.067296013801, 7661.06723205933, 7660.71819198431, 7660.212421103769, 7659.9636479664405, 7659.938975524531, 7660.061957987962, 7660.037023111568, 7659.887733518575, 7659.93688853576, 7660.035261841736, 7660.059807712877, 7660.157134486219, 7660.358131730846, 7660.456505021519, 7660.688569495339, 7660.782609598131, 7660.7621629839505], "e_w_deviation": [0.0, -1.484000657881275e-17, -2.175842355175559, -2.2419840214828732, -2.593877605762808, -3.389295211600157, -4.0542389625514135, -4.027180204159597, -4.13311241136453, -4.645452673436392, -4.92810207452572, -5.146432756144027, -5.3339936813724895, -5.721190851580817, -6.269858859107042, -6.660334283164571, -7.3815342656473435, -8.37630368630489, -8.922884580145645, -9.340341250202624, -8.881544780257427, -8.643856233265076, -9.568723135321147, -10.156517109805641, -10.386186489246885, -10.682769333247977, -10.989832648807775, -11.56584727461761, -11.326072883057673, -11.194436173167277, -12.848022933081339, -14.74132409432717, -16.436352123638066, -18.267824737470026, -20.16774917889891, -22.018285039135044, -23.25527466581436, -24.726300840835037, -26.61839395085467, -28.188184254357466, -29.70970162039467, -31.202535397595472, -32.6585055963508, -33.99637609819523, -35.29196955641501, -36.43259059281338, -37.076884648839126, -37.28718688470833, -37.179510885615926, -36.772341260215114, -36.07404142749863, -36.23950271973721, -36.46852254598554, -36.17970824196029, -35.79751817472034, -34.946266913548605, -35.366680506690855, -35.39092003219164, -33.91681798193058, -32.48536824485758, -30.345299422455874, -22.023784850465717, -6.26921560023823, 16.190843339757308, 44.10392321003876, 80.4614298575519, 122.79065099746501, 171.4101943000012, 221.13581267417885, 274.1324990599122, 328.4511281163804, 386.6108371411615, 445.11552106990206, 505.050118137853, 567.5859208385572, 630.4085035962628, 696.3781907690641, 761.4882661350741, 829.1884923449869, 897.273922021971, 967.4149370948395, 1037.8354016688197, 1108.6904588935624, 1178.8053576711623, 1247.9186329320553, 1317.143065996658, 1365.4641853124037, 1414.059585780118, 1482.4277171087615, 1547.5117843566725, 1610.1272775774444, 1666.1732919750543, 1727.121526626948, 1788.0380737677126, 1846.657962591831, 1906.9715579444687, 1967.2145995930707, 2024.0824721238525, 2077.6932327252953, 2129.310672394236, 2178.966423288364, 2226.4320834376203, 2272.382292878736, 2317.4584385834237, 2362.4098278862716, 2408.116276072377, 2450.9945672173526, 2493.6871237531554, 2515.841019107563, 2533.664749805278], "n_s_deviation": [0.0, -1.3785312281415713e-17, -2.0212030353173684, -2.117474233940387, -2.5433114844573717, -2.9746580295078346, -3.096872330184516, -3.547025241268128, -4.244318643030875, -5.212363072305503, -5.416670419759379, -5.6644522515964795, -7.4343096514447415, -9.524363563889594, -11.617315201593373, -13.657874309319288, -15.570720570759654, -17.313276242698638, -19.147666498237268, -21.1092737561569, -22.343018956190242, -23.278903111169665, -24.92449035064133, -26.58983984591266, -28.010791491809098, -29.560178344151375, -31.14334036107004, -32.880100514039235, -34.46108331932539, -35.79161560995158, -36.98033508016032, -37.81162348161602, -38.70783391216995, -39.90527839354168, -41.31250151308184, -42.851066051697146, -44.616523833118926, -44.74664698463293, -45.07464567742085, -47.55036106024729, -50.255575155570206, -53.0082836249512, -55.85620121029757, -58.59001671264978, -61.00413274169478, -63.432814219688424, -65.64517228195949, -67.44747737684362, -69.05271904531232, -70.56045471772394, -71.8677961030382, -72.60733839941273, -73.17719626309531, -74.33622436740495, -75.76491253054486, -76.87146038506376, -76.76731390609072, -76.48908622388853, -77.16224878848631, -77.51592653796689, -76.26931984432156, -69.42301100110615, -56.12611778437354, -37.30449166745683, -13.071349447776281, 18.671608779555633, 55.903969746441945, 98.68653026453202, 141.5586353467743, 186.6204852148685, 232.68513445656907, 281.9114267345113, 331.9574329881619, 383.69922686653683, 437.3427265322022, 491.3640061689003, 548.9693831616727, 606.1482488956053, 665.3941282074068, 724.7349560515827, 785.1583975473234, 845.2603395856305, 905.9484648652372, 968.0811468556537, 1031.5898084053179, 1095.1221714720284, 1138.5924821915255, 1181.7602222204512, 1246.2095976634116, 1314.0074970566761, 1384.100598108905, 1449.3291138949253, 1520.8923767738684, 1593.786477219505, 1665.9825226964995, 1739.3702604404925, 1812.8157838142843, 1887.627886485367, 1964.8210992507668, 2044.5636846900316, 2125.546868561508, 2206.677019325788, 2288.6766183469813, 2371.1635761844777, 2452.576044537638, 2536.9850257909825, 2620.632461288653, 2705.4986688433305, 2749.2041631671486, 2783.892820441016], "dls": [0.0, 0.0, 0.062178175697026876, 1.312323104221892, 0.43922131729609876, 0.08042287727355951, 0.20007154008744296, 0.30541848464608146, 0.07544251812726605, 0.2976066556324211, 0.23670095082420844, 0.24213627593834536, 0.2999850410059419, 0.09982823459047929, 0.06001822909970222, 0.03037855739338735, 0.056789950199735574, 0.17042476568489642, 0.0693668431679535, 0.01993292540609921, 0.3157502687404893, 0.0720972968028324, 0.26974508589212887, 0.40057331440554544, 0.009985005553010146, 0.14992993001118987, 0.11006274453029032, 0.37915058010860575, 0.2072590379355382, 0.009102498691501362, 0.26684825484593366, 0.3100147696535474, 0.1398957617883856, 0.16989850673084672, 0.029979119030003634, 0.019904290966461985, 0.23348528080380748, 0.40804615992287036, 0.07652363332976642, 0.19998126332911942, 0.00017422010087074383, 0.029934816672579877, 0.04973451056650544, 0.23010514458114228, 0.1200014784499837, 0.059735768555311125, 0.4808912031722217, 0.10002129508581828, 0.11085278275925033, 0.029990726732150582, 0.08124578232425676, 0.07575783435644577, 0.14302641690198467, 0.26538522507724854, 0.02519933025313245, 0.28000418182841885, 0.4054270579062658, 0.21400934683013106, 0.039905124947546486, 0.15180963459746707, 8.09081900129934, 6.6802380356223, 5.297841217404203, 5.671609243355286, 5.934962274534154, 8.374925113240641, 6.676388904579787, 4.155341460623003, 1.0299674900525506, 3.5683840146139207, 2.591380087028445, 2.8124879045508773, 2.3485158500356245, 2.7552416559590474, 3.083781392084175, 2.9666693510617885, 3.0398535258486246, 3.0643100952872393, 2.357883414036999, 3.276289102066852, 3.366497713956813, 2.968055033124839, 3.3291719989157795, 1.6870498749295495, 1.541073533810662, 1.5724205308300976, 1.7478215994323207, 0.8461880746380327, 0.08166466134158418, 0.07940585015175593, 0.0008274675158041158, 0.024721092669823027, 0.09587844093486547, 0.11802576377694084, 0.32912147949062376, 0.15358234453355382, 0.20905337189170117, 0.16747860550934596, 0.13825524436433095, 0.26098935876969753, 0.1397644970882708, 0.14015134747360147, 0.0550950458378559, 0.031334689863031874, 0.13431049244001467, 0.025960717101329234, 0.12014006714273541, 0.29474534107044165, 0.6920544308284147, 0.15599660612029712], "surface_latitude": 29.90829444, "surface_longitude": 47.68852083, "longitude_points": [47.688520831290745, 47.688520831290745, 47.688513819700496, 47.688513603988845, 47.688512462714655, 47.68850992221447, 47.68850781604027, 47.68850786813882, 47.688507482550484, 47.68850579520865, 47.688504888680896, 47.68850418179598, 47.68850345955801, 47.68850208404778, 47.688500199065075, 47.68849881687097, 47.688496401044404, 47.68849313498969, 47.68849127568371, 47.68848981422514, 47.68849117010627, 47.68849185063625, 47.68848881220671, 47.688486835403225, 47.68848600612662, 47.68848495632938, 47.68848387098408, 47.68848192605856, 47.68848256553063, 47.688482882444475, 47.688477579464184, 47.688471546849684, 47.68846613476939, 47.68846027011657, 47.688454174085145, 47.68844822412082, 47.688444192448486, 47.68843954341255, 47.688433551774125, 47.68842841804527, 47.688423419617074, 47.6884185081469, 47.68841370591006, 47.68840928457057, 47.68840502017516, 47.68840124346762, 47.68839904808479, 47.688398251734604, 47.68839847279771, 47.6883996456245, 47.68840175143515, 47.68840117498318, 47.68840041060566, 47.6884012359041, 47.68840233578538, 47.68840493881123, 47.68840362057228, 47.68840356466866, 47.688408164085736, 47.688412652574065, 47.688419494121405, 47.688446244633305, 47.68849691437695, 47.68856914038612, 47.68865896423556, 47.68877597548383, 47.68891222641433, 47.689068726484834, 47.689228721956944, 47.6893991961312, 47.68957391405658, 47.68976098035547, 47.6899491957213, 47.69014204669679, 47.690343242692066, 47.690545371654714, 47.69075769179644, 47.6909672699112, 47.6911851705984, 47.69140429389128, 47.69162998109378, 47.691856526609016, 47.6920844868699, 47.69231022027474, 47.69253289712188, 47.6927559270598, 47.6929115454283, 47.69306800684372, 47.693288405669634, 47.6934986951688, 47.69370136944729, 47.69388296446839, 47.69408049051037, 47.694278015881174, 47.69446824670402, 47.69466390845428, 47.69485935273808, 47.695044253643516, 47.69521905861008, 47.69538776611093, 47.69555037899606, 47.69570609586238, 47.69585709802761, 47.6960053801436, 47.696153189869406, 47.696303603761564, 47.69644504173073, 47.69658598484674, 47.6966590982265, 47.69671788597802], "latitude_points": [29.90829449401083, 29.90829449401083, 29.90828908033187, 29.908288820062644, 29.90828767262826, 29.908286538589756, 29.908286245560088, 29.90828500693392, 29.908283097795564, 29.908280470860387, 29.908279927668985, 29.908279260884825, 29.90827440991334, 29.90826869198113, 29.908262976474855, 29.908257394750386, 29.908252185219315, 29.908247461195472, 29.908242456003986, 29.908237092951193, 29.908233673472793, 29.908231086652023, 29.90822662457114, 29.908222086504303, 29.90821819693012, 29.908213958759212, 29.908209628458504, 29.908204893418652, 29.908200533925786, 29.908196869552825, 29.9081937096884, 29.908191547358893, 29.908189193887285, 29.908186021492305, 29.908182277091846, 29.908178168626872, 29.908173397255972, 29.90817313435112, 29.908172354831493, 29.908165653294517, 29.908158318058035, 29.908150850475728, 29.908143118915522, 29.90813569327592, 29.90812914335489, 29.9081225434426, 29.90811650599286, 29.908111567326724, 29.90810714967598, 29.90810298067576, 29.908099343570292, 29.908097322173667, 29.908095771107895, 29.90809256786567, 29.908088617671282, 29.90808552244678, 29.908085835655896, 29.908086601701672, 29.908084657221394, 29.908083593333533, 29.908086880961676, 29.908105157229674, 29.908140679572952, 29.908190950806258, 29.908255740385076, 29.908340621298073, 29.908440201079728, 29.908554626212, 29.90866922599756, 29.908789631808254, 29.908912707679214, 29.909044223294977, 29.909177968731562, 29.90931628132409, 29.909459651441434, 29.909604040772667, 29.909758075116038, 29.90991099250654, 29.910069422231054, 29.910228087628404, 29.91038959489801, 29.910550200348887, 29.910712388013714, 29.910878592118223, 29.911048641091558, 29.911218747598728, 29.911335076533952, 29.91145055621365, 29.911623236550348, 29.911805328857348, 29.91199388641097, 29.912169500782085, 29.91236220468478, 29.91255856700546, 29.91275315904239, 29.91295091592016, 29.913148835780984, 29.913350727925835, 29.913559372395248, 29.913775150036457, 29.91399446273615, 29.914214320302598, 29.91443666438243, 29.914660403746986, 29.914881198593413, 29.915110177958542, 29.915337247141355, 29.915567676907617, 29.91568633571068, 29.915780498580656], "zone_number": 38, "zone_letter": "R", "x_points": [759587.9344401711, 759587.9344401711, 759587.2712434212, 759587.2510834413, 759587.1438262769, 759586.9013829906, 759586.6987081353, 759586.7069556449, 759586.6746675081, 759586.5185061962, 759586.4323546588, 759586.365807467, 759586.308638897, 759586.1906211996, 759586.0233871909, 759585.9043702816, 759585.6845485269, 759585.3813428076, 759585.2147449511, 759585.087504158, 759585.2273453221, 759585.2997927913, 759585.0178933594, 759584.8387337561, 759584.7687305292, 759584.6783320784, 759584.5847391797, 759584.4091699218, 759584.4822531564, 759584.5223760256, 759584.0183627811, 759583.4412845871, 759582.9246400439, 759582.3664071911, 759581.7873102213, 759581.2232668912, 759580.846232453, 759580.3978636748, 759579.8211536949, 759579.3426816104, 759578.8789231172, 759578.423907382, 759577.9801276653, 759577.5723447364, 759577.1774478504, 759576.8297865584, 759576.6334057301, 759576.5693056086, 759576.6021252532, 759576.726230555, 759576.939072344, 759576.8886397422, 759576.8188344991, 759576.906865099, 759577.0233566315, 759577.2828180159, 759577.1546759526, 759577.1472877453, 759577.5965940502, 759578.03289993, 759578.6851929071, 759581.2215905486, 759586.0235832562, 759592.8694092211, 759601.3773159655, 759612.4590839917, 759625.3610305951, 759640.1802673937, 759655.3366358742, 759671.4900258846, 759688.046344021, 759705.7734233318, 759723.6056509932, 759741.8737161795, 759760.9346288427, 759780.0829520673, 759800.1905127175, 759820.036063689, 759840.6710926378, 759861.4235316034, 759882.8025129976, 759904.2666705998, 759925.8632920418, 759947.2343131893, 759968.3000394888, 759989.3996466869, 760004.1279238543, 760018.939801917, 760039.7784083459, 760059.616032043, 760078.7012343768, 760095.7840595652, 760114.361081487, 760132.9284450555, 760150.7957871691, 760169.1793710326, 760187.541450127, 760204.8747776744, 760221.2153375058, 760236.9483331168, 760252.0834059895, 760266.5509392029, 760280.5565630406, 760294.2957722513, 760307.9969557108, 760321.9282811179, 760334.9975842589, 760348.010275491, 760354.7627827951, 760360.1954559118], "y_points": [3311661.864849136, 3311661.864849136, 3311661.248786451, 3311661.2194429897, 3311661.0896477955, 3311660.9581733686, 3311660.92092245, 3311660.7837158428, 3311660.571180814, 3311660.276120872, 3311660.2138479925, 3311660.13832409, 3311659.5988715542, 3311658.961823122, 3311658.3238914628, 3311657.701929047, 3311657.1188935065, 3311656.5877625374, 3311656.0286403876, 3311655.430742495, 3311655.0546969585, 3311654.7694394677, 3311654.2678644774, 3311653.760265951, 3311653.3271598895, 3311652.854906777, 3311652.372358994, 3311651.8429944995, 3311651.3611109406, 3311650.955564698, 3311650.593243004, 3311650.339866299, 3311650.0667013596, 3311649.701720282, 3311649.272798675, 3311648.8038442037, 3311648.265732672, 3311648.226071135, 3311648.126097134, 3311647.371499085, 3311646.546949829, 3311645.7079242873, 3311644.8398790075, 3311644.006612042, 3311643.2707894766, 3311642.530527362, 3311641.8562006247, 3311641.3068580315, 3311640.817580371, 3311640.3580225385, 3311639.959544884, 3311639.734132392, 3311639.560439715, 3311639.207167949, 3311638.771703797, 3311638.434428011, 3311638.4661718574, 3311638.550975655, 3311638.3457957054, 3311638.237994727, 3311638.6179604474, 3311640.7047153832, 3311644.7576084356, 3311650.494440076, 3311657.8807018246, 3311667.5559554924, 3311678.904379115, 3311691.9445035607, 3311705.0119211897, 3311718.7467730297, 3311732.7872781185, 3311747.791452005, 3311763.045474711, 3311778.816373485, 3311795.166912183, 3311811.6325982166, 3311829.190717124, 3311846.6188353994, 3311864.6769794137, 3311882.7640637406, 3311901.1811287086, 3311919.500200642, 3311937.997941227, 3311956.9359826976, 3311976.293422738, 3311995.6580870007, 3312008.907837708, 3312022.065364869, 3312041.709534504, 3312062.374334239, 3312083.73871144, 3312103.6203630515, 3312125.4328455767, 3312147.6509673926, 3312169.656322054, 3312192.0249045184, 3312214.411100043, 3312237.213828937, 3312260.742320188, 3312285.04786023, 3312309.731534674, 3312334.460004627, 3312359.4534824085, 3312384.595507157, 3312409.4100275114, 3312435.1378849973, 3312460.633623337, 3312486.5008433997, 3312499.8222780693, 3312510.3953808066], "surface_x": 759587.9344401711, "surface_y": 3311661.864849136, "isHorizontal": ["Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal"]}'
Now you have done it, you can check out your calculated points in a dataframe for easy viewing.
[8]:
json_ds_obj = json.loads(json_ds)
df = pd.DataFrame(json_ds_obj)
df.head()
[8]:
wellId | md | inc | azim | tvd | e_w_deviation | n_s_deviation | dls | surface_latitude | surface_longitude | longitude_points | latitude_points | zone_number | zone_letter | x_points | y_points | surface_x | surface_y | isHorizontal | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | well_C | 0.00 | 0.00 | 227.11 | 0.000000 | 0.000000e+00 | 0.000000e+00 | 0.000000 | 29.908294 | 47.688521 | 47.688521 | 29.908294 | 38 | R | 759587.934440 | 3.311662e+06 | 759587.93444 | 3.311662e+06 | Vertical |
1 | well_C | 35.00 | 0.00 | 227.11 | 35.000000 | -1.484001e-17 | -1.378531e-17 | 0.000000 | 29.908294 | 47.688521 | 47.688521 | 29.908294 | 38 | R | 759587.934440 | 3.311662e+06 | 759587.93444 | 3.311662e+06 | Vertical |
2 | well_C | 774.81 | 0.46 | 227.11 | 774.802052 | -2.175842e+00 | -2.021203e+00 | 0.062178 | 29.908294 | 47.688521 | 47.688514 | 29.908289 | 38 | R | 759587.271243 | 3.311661e+06 | 759587.93444 | 3.311662e+06 | Vertical |
3 | well_C | 800.00 | 0.13 | 163.86 | 799.991684 | -2.241984e+00 | -2.117474e+00 | 1.312323 | 29.908294 | 47.688521 | 47.688514 | 29.908289 | 38 | R | 759587.251083 | 3.311661e+06 | 759587.93444 | 3.311662e+06 | Vertical |
4 | well_C | 900.00 | 0.57 | 230.43 | 899.989571 | -2.593878e+00 | -2.543311e+00 | 0.439221 | 29.908294 | 47.688521 | 47.688512 | 29.908288 | 38 | R | 759587.143826 | 3.311661e+06 | 759587.93444 | 3.311662e+06 | Vertical |
[9]:
df.plot(kind="scatter", x="longitude_points", y="latitude_points", alpha=0.4)
plt.show()
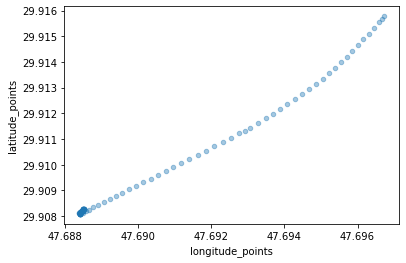
[10]:
fig = pyplot.figure()
ax = Axes3D(fig)
ax.scatter(df['longitude_points'].values, df['latitude_points'].values, df['tvd'].values*-1)
pyplot.show()
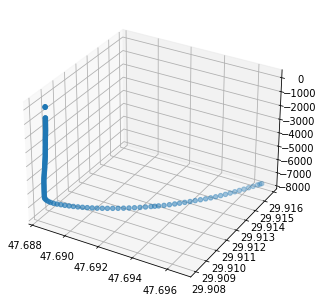
[11]:
# Finished
Calculate From WellId, MD, INC, AZIM and Suface X,Y¶
Sometimes the latitue and longitude coordinates are not provided.
In the case that only X,Y surface coordinates are provided you can still calculate the survey points with one additional step.
The user must find the CRS coordinate system and provide that in the calculation.
Transformation Tested with latitude and longitude points error less than 0.001%
[12]:
# get data that has only surface x and y, wellid, md, inc, azim
json_path = './data/well_export.json'
[13]:
with open(json_path) as json_file:
json_obj = json.load(json_file)
json_file.close()
#create a dict that has only the surface x and y
well_dict = {'wellId':json_obj['wellId'],
'md':json_obj['md'],
'inc':json_obj['inc'],
'azim':json_obj['azim'],
'surface_x':json_obj['surface_x'],
'surface_y':json_obj['surface_y']}
#well_dict
Since we already have a dictionary created, we do not need the from_json
Enter the dict into the WellboreTrajectory(data)
[14]:
# import dict data
well_obj = WellboreTrajectory(data = well_dict)
well_obj.deviation_survey_obj
[14]:
DeviationSurvey(wellId='well_C', md=array([ 0. , 35. , 774.81, 800. , 900. , 1000. ,
1100. , 1200. , 1300. , 1400. , 1500. , 1600. ,
1700. , 1800. , 1900. , 2000. , 2100. , 2200. ,
2300. , 2400. , 2500. , 2600. , 2700. , 2800. ,
2900. , 3000. , 3100. , 3200. , 3300. , 3400. ,
3500. , 3600. , 3700. , 3800. , 3900. , 4000. ,
4100. , 4200. , 4300. , 4400. , 4500. , 4600. ,
4700. , 4800. , 4900. , 5000. , 5100. , 5200. ,
5300. , 5400. , 5500. , 5600. , 5700. , 5800. ,
5900. , 6000. , 6100. , 6200. , 6300. , 6400. ,
6450.67, 6532. , 6625. , 6720. , 6813. , 6909. ,
7002. , 7098. , 7191. , 7286. , 7379. , 7475. ,
7569. , 7663. , 7758. , 7851. , 7947. , 8040. ,
8135. , 8229. , 8324. , 8418. , 8512. , 8606. ,
8700. , 8794. , 8859. , 8924. , 9018. , 9112. ,
9206. , 9292. , 9386. , 9481. , 9574. , 9669. ,
9764. , 9858. , 9952. , 10047. , 10142. , 10236. ,
10330. , 10424. , 10517. , 10613. , 10707. , 10802. ,
10851. , 10890. ]), inc=array([ 0. , 0. , 0.46, 0.13, 0.57, 0.49, 0.29, 0.6 , 0.53,
0.83, 0.61, 0.87, 1.17, 1.27, 1.21, 1.18, 1.24, 1.07,
1.14, 1.16, 0.86, 0.95, 1.22, 0.82, 0.83, 0.98, 0.87,
1.25, 1.06, 1.07, 1.34, 1.03, 1.17, 1.34, 1.37, 1.39,
1.16, 1.61, 1.58, 1.78, 1.78, 1.81, 1.86, 1.63, 1.51,
1.57, 1.09, 0.99, 0.88, 0.91, 0.83, 0.77, 0.64, 0.91,
0.94, 0.66, 1.09, 0.91, 0.95, 0.8 , 4.91, 10.35, 15.28,
20.67, 26.19, 34.23, 40.44, 44.43, 45.39, 48.78, 51.19, 53.89,
56.1 , 58.69, 61.62, 64.38, 67.3 , 70.15, 72.39, 75.47, 78.67,
81.46, 84.59, 86.2 , 87.65, 89.13, 90.27, 89.72, 89.9 , 90.02,
90.05, 90.03, 89.94, 90.06, 90.37, 90.24, 90.06, 89.97, 89.88,
90.15, 90.03, 89.91, 89.97, 90. , 89.88, 89.88, 90. , 89.72,
90.06, 90. ]), azim=array([227.11, 227.11, 227.11, 163.86, 230.43, 254.47, 268.25, 147.67,
236.56, 190.05, 342.93, 184.69, 187.06, 193.66, 195.77, 185.77,
214.81, 203.82, 189.81, 194.18, 109.65, 214.46, 205.35, 190.63,
187.75, 193.45, 188.19, 205.4 , 130.09, 218.09, 247.16, 245.17,
239.46, 234.52, 232.45, 228.1 , 199.28, 305.98, 213.22, 211.63,
207.08, 209.84, 204.39, 208. , 208.46, 201.98, 187.95, 185.23,
165.95, 163.86, 138.75, 253.13, 132.16, 189.07, 141.79, 143.34,
306.53, 111.26, 117.69, 87.41, 55.44, 48.23, 50.93, 49.37,
48.77, 48.96, 48.41, 48.88, 49.58, 49.67, 49.73, 49.78,
49.14, 49.25, 49.5 , 49.12, 48.63, 48.79, 48.83, 49.02,
49.49, 49.55, 49.29, 47.62, 47.22, 47.69, 48.36, 48.41,
44.97, 42.69, 40.86, 40.48, 40.36, 39.41, 38.74, 40.09,
38.63, 35.85, 33.71, 32.12, 30.91, 29.75, 28.78, 28.53,
29.28, 27.59, 26.69, 26.72, 27.04, 27.35]), surface_latitude=None, surface_longitude=None, tvd=None, n_s_deviation=None, e_w_deviation=None, dls=None, surface_x=759587.9344401711, surface_y=3311661.864849136, x_points=None, y_points=None, zone_number=None, zone_letter=None, latitude_points=None, longitude_points=None, isHorizontal=None)
As you can see above, no surface latitude and longitude are provided. Only the surface X and Y.
CRS Transformation: Since we are only given surface x and y we must translate this to the WGS:84 projection system. To do this use crs_transform()
and enter in the EPSG coordinate system.
Find your CRS here: https://epsg.io/
[15]:
# must import crs transform string
well_obj.crs_transform(crs_in='epsg:32638')
Calculate Survey Points:
[16]:
well_obj.calculate_survey_points()
# check out object
well_obj.deviation_survey_obj
[16]:
DeviationSurvey(wellId='well_C', md=array([ 0. , 35. , 774.81, 800. , 900. , 1000. ,
1100. , 1200. , 1300. , 1400. , 1500. , 1600. ,
1700. , 1800. , 1900. , 2000. , 2100. , 2200. ,
2300. , 2400. , 2500. , 2600. , 2700. , 2800. ,
2900. , 3000. , 3100. , 3200. , 3300. , 3400. ,
3500. , 3600. , 3700. , 3800. , 3900. , 4000. ,
4100. , 4200. , 4300. , 4400. , 4500. , 4600. ,
4700. , 4800. , 4900. , 5000. , 5100. , 5200. ,
5300. , 5400. , 5500. , 5600. , 5700. , 5800. ,
5900. , 6000. , 6100. , 6200. , 6300. , 6400. ,
6450.67, 6532. , 6625. , 6720. , 6813. , 6909. ,
7002. , 7098. , 7191. , 7286. , 7379. , 7475. ,
7569. , 7663. , 7758. , 7851. , 7947. , 8040. ,
8135. , 8229. , 8324. , 8418. , 8512. , 8606. ,
8700. , 8794. , 8859. , 8924. , 9018. , 9112. ,
9206. , 9292. , 9386. , 9481. , 9574. , 9669. ,
9764. , 9858. , 9952. , 10047. , 10142. , 10236. ,
10330. , 10424. , 10517. , 10613. , 10707. , 10802. ,
10851. , 10890. ]), inc=array([ 0. , 0. , 0.46, 0.13, 0.57, 0.49, 0.29, 0.6 , 0.53,
0.83, 0.61, 0.87, 1.17, 1.27, 1.21, 1.18, 1.24, 1.07,
1.14, 1.16, 0.86, 0.95, 1.22, 0.82, 0.83, 0.98, 0.87,
1.25, 1.06, 1.07, 1.34, 1.03, 1.17, 1.34, 1.37, 1.39,
1.16, 1.61, 1.58, 1.78, 1.78, 1.81, 1.86, 1.63, 1.51,
1.57, 1.09, 0.99, 0.88, 0.91, 0.83, 0.77, 0.64, 0.91,
0.94, 0.66, 1.09, 0.91, 0.95, 0.8 , 4.91, 10.35, 15.28,
20.67, 26.19, 34.23, 40.44, 44.43, 45.39, 48.78, 51.19, 53.89,
56.1 , 58.69, 61.62, 64.38, 67.3 , 70.15, 72.39, 75.47, 78.67,
81.46, 84.59, 86.2 , 87.65, 89.13, 90.27, 89.72, 89.9 , 90.02,
90.05, 90.03, 89.94, 90.06, 90.37, 90.24, 90.06, 89.97, 89.88,
90.15, 90.03, 89.91, 89.97, 90. , 89.88, 89.88, 90. , 89.72,
90.06, 90. ]), azim=array([227.11, 227.11, 227.11, 163.86, 230.43, 254.47, 268.25, 147.67,
236.56, 190.05, 342.93, 184.69, 187.06, 193.66, 195.77, 185.77,
214.81, 203.82, 189.81, 194.18, 109.65, 214.46, 205.35, 190.63,
187.75, 193.45, 188.19, 205.4 , 130.09, 218.09, 247.16, 245.17,
239.46, 234.52, 232.45, 228.1 , 199.28, 305.98, 213.22, 211.63,
207.08, 209.84, 204.39, 208. , 208.46, 201.98, 187.95, 185.23,
165.95, 163.86, 138.75, 253.13, 132.16, 189.07, 141.79, 143.34,
306.53, 111.26, 117.69, 87.41, 55.44, 48.23, 50.93, 49.37,
48.77, 48.96, 48.41, 48.88, 49.58, 49.67, 49.73, 49.78,
49.14, 49.25, 49.5 , 49.12, 48.63, 48.79, 48.83, 49.02,
49.49, 49.55, 49.29, 47.62, 47.22, 47.69, 48.36, 48.41,
44.97, 42.69, 40.86, 40.48, 40.36, 39.41, 38.74, 40.09,
38.63, 35.85, 33.71, 32.12, 30.91, 29.75, 28.78, 28.53,
29.28, 27.59, 26.69, 26.72, 27.04, 27.35]), surface_latitude=29.90829443997491, surface_longitude=47.68852083021084, tvd=array([ 0. , 35. , 774.80205236, 799.9916839 ,
899.98957066, 999.98528439, 1099.98291708, 1199.97977187,
1299.97490562, 1399.96774509, 1499.95980744, 1599.95135856,
1699.93539853, 1799.91271696, 1899.88929431, 1999.86754393,
2099.8452397 , 2199.8248857 , 2299.80628255, 2399.78613986,
2499.77051364, 2599.75802178, 2699.73999928, 2799.7239516 ,
2899.71358513, 2999.70108231, 3099.68803535, 3199.67073753,
3299.65039143, 3399.63311651, 3499.6109049 , 3599.58939615,
3699.5709424 , 3799.54691757, 3899.51895345, 3999.48994863,
4099.46512692, 4199.43556378, 4299.39682997, 4399.35379463,
4499.30554098, 4599.25646956, 4699.20518489, 4799.15874306,
4899.12118453, 4999.0850605 , 5099.05782961, 5199.04134366,
5299.02801386, 5399.01581265, 5499.004277 , 5598.99453025,
5698.9869478 , 5798.9777011 , 5898.96466764, 5998.95482054,
6098.94287286, 6198.92763525, 6298.9144603 , 6398.90277221,
6449.49892068, 6530.07840696, 6620.73385719, 6711.06365715,
6796.36247083, 6879.25635883, 6953.16472562, 7024.00253299,
7089.86588017, 7154.54311968, 7214.33658158, 7272.71906108,
7326.6385766 , 7377.28559394, 7424.55766084, 7466.7746503 ,
7506.06181579, 7539.80284576, 7570.306201 , 7596.32330294,
7617.57772512, 7633.79396604, 7645.20749536, 7652.75404791,
7657.79630452, 7660.43720229, 7660.77749063, 7660.78311918,
7661.09477093, 7661.16033202, 7661.10284739, 7661.04275014,
7661.06729601, 7661.06723206, 7660.71819198, 7660.2124211 ,
7659.96364797, 7659.93897552, 7660.06195799, 7660.03702311,
7659.88773352, 7659.93688854, 7660.03526184, 7660.05980771,
7660.15713449, 7660.35813173, 7660.45650502, 7660.6885695 ,
7660.7826096 , 7660.76216298]), n_s_deviation=array([ 0.00000000e+00, -1.37853123e-17, -2.02120304e+00, -2.11747423e+00,
-2.54331148e+00, -2.97465803e+00, -3.09687233e+00, -3.54702524e+00,
-4.24431864e+00, -5.21236307e+00, -5.41667042e+00, -5.66445225e+00,
-7.43430965e+00, -9.52436356e+00, -1.16173152e+01, -1.36578743e+01,
-1.55707206e+01, -1.73132762e+01, -1.91476665e+01, -2.11092738e+01,
-2.23430190e+01, -2.32789031e+01, -2.49244904e+01, -2.65898398e+01,
-2.80107915e+01, -2.95601783e+01, -3.11433404e+01, -3.28801005e+01,
-3.44610833e+01, -3.57916156e+01, -3.69803351e+01, -3.78116235e+01,
-3.87078339e+01, -3.99052784e+01, -4.13125015e+01, -4.28510661e+01,
-4.46165238e+01, -4.47466470e+01, -4.50746457e+01, -4.75503611e+01,
-5.02555752e+01, -5.30082836e+01, -5.58562012e+01, -5.85900167e+01,
-6.10041327e+01, -6.34328142e+01, -6.56451723e+01, -6.74474774e+01,
-6.90527190e+01, -7.05604547e+01, -7.18677961e+01, -7.26073384e+01,
-7.31771963e+01, -7.43362244e+01, -7.57649125e+01, -7.68714604e+01,
-7.67673139e+01, -7.64890862e+01, -7.71622488e+01, -7.75159265e+01,
-7.62693198e+01, -6.94230110e+01, -5.61261178e+01, -3.73044917e+01,
-1.30713494e+01, 1.86716088e+01, 5.59039697e+01, 9.86865303e+01,
1.41558635e+02, 1.86620485e+02, 2.32685134e+02, 2.81911427e+02,
3.31957433e+02, 3.83699227e+02, 4.37342727e+02, 4.91364006e+02,
5.48969383e+02, 6.06148249e+02, 6.65394128e+02, 7.24734956e+02,
7.85158398e+02, 8.45260340e+02, 9.05948465e+02, 9.68081147e+02,
1.03158981e+03, 1.09512217e+03, 1.13859248e+03, 1.18176022e+03,
1.24620960e+03, 1.31400750e+03, 1.38410060e+03, 1.44932911e+03,
1.52089238e+03, 1.59378648e+03, 1.66598252e+03, 1.73937026e+03,
1.81281578e+03, 1.88762789e+03, 1.96482110e+03, 2.04456368e+03,
2.12554687e+03, 2.20667702e+03, 2.28867662e+03, 2.37116358e+03,
2.45257604e+03, 2.53698503e+03, 2.62063246e+03, 2.70549867e+03,
2.74920416e+03, 2.78389282e+03]), e_w_deviation=array([ 0.00000000e+00, -1.48400066e-17, -2.17584236e+00, -2.24198402e+00,
-2.59387761e+00, -3.38929521e+00, -4.05423896e+00, -4.02718020e+00,
-4.13311241e+00, -4.64545267e+00, -4.92810207e+00, -5.14643276e+00,
-5.33399368e+00, -5.72119085e+00, -6.26985886e+00, -6.66033428e+00,
-7.38153427e+00, -8.37630369e+00, -8.92288458e+00, -9.34034125e+00,
-8.88154478e+00, -8.64385623e+00, -9.56872314e+00, -1.01565171e+01,
-1.03861865e+01, -1.06827693e+01, -1.09898326e+01, -1.15658473e+01,
-1.13260729e+01, -1.11944362e+01, -1.28480229e+01, -1.47413241e+01,
-1.64363521e+01, -1.82678247e+01, -2.01677492e+01, -2.20182850e+01,
-2.32552747e+01, -2.47263008e+01, -2.66183940e+01, -2.81881843e+01,
-2.97097016e+01, -3.12025354e+01, -3.26585056e+01, -3.39963761e+01,
-3.52919696e+01, -3.64325906e+01, -3.70768846e+01, -3.72871869e+01,
-3.71795109e+01, -3.67723413e+01, -3.60740414e+01, -3.62395027e+01,
-3.64685225e+01, -3.61797082e+01, -3.57975182e+01, -3.49462669e+01,
-3.53666805e+01, -3.53909200e+01, -3.39168180e+01, -3.24853682e+01,
-3.03452994e+01, -2.20237849e+01, -6.26921560e+00, 1.61908433e+01,
4.41039232e+01, 8.04614299e+01, 1.22790651e+02, 1.71410194e+02,
2.21135813e+02, 2.74132499e+02, 3.28451128e+02, 3.86610837e+02,
4.45115521e+02, 5.05050118e+02, 5.67585921e+02, 6.30408504e+02,
6.96378191e+02, 7.61488266e+02, 8.29188492e+02, 8.97273922e+02,
9.67414937e+02, 1.03783540e+03, 1.10869046e+03, 1.17880536e+03,
1.24791863e+03, 1.31714307e+03, 1.36546419e+03, 1.41405959e+03,
1.48242772e+03, 1.54751178e+03, 1.61012728e+03, 1.66617329e+03,
1.72712153e+03, 1.78803807e+03, 1.84665796e+03, 1.90697156e+03,
1.96721460e+03, 2.02408247e+03, 2.07769323e+03, 2.12931067e+03,
2.17896642e+03, 2.22643208e+03, 2.27238229e+03, 2.31745844e+03,
2.36240983e+03, 2.40811628e+03, 2.45099457e+03, 2.49368712e+03,
2.51584102e+03, 2.53366475e+03]), dls=array([0.00000000e+00, 0.00000000e+00, 6.21781757e-02, 1.31232310e+00,
4.39221317e-01, 8.04228773e-02, 2.00071540e-01, 3.05418485e-01,
7.54425181e-02, 2.97606656e-01, 2.36700951e-01, 2.42136276e-01,
2.99985041e-01, 9.98282346e-02, 6.00182291e-02, 3.03785574e-02,
5.67899502e-02, 1.70424766e-01, 6.93668432e-02, 1.99329254e-02,
3.15750269e-01, 7.20972968e-02, 2.69745086e-01, 4.00573314e-01,
9.98500555e-03, 1.49929930e-01, 1.10062745e-01, 3.79150580e-01,
2.07259038e-01, 9.10249869e-03, 2.66848255e-01, 3.10014770e-01,
1.39895762e-01, 1.69898507e-01, 2.99791190e-02, 1.99042910e-02,
2.33485281e-01, 4.08046160e-01, 7.65236333e-02, 1.99981263e-01,
1.74220101e-04, 2.99348167e-02, 4.97345106e-02, 2.30105145e-01,
1.20001478e-01, 5.97357686e-02, 4.80891203e-01, 1.00021295e-01,
1.10852783e-01, 2.99907267e-02, 8.12457823e-02, 7.57578344e-02,
1.43026417e-01, 2.65385225e-01, 2.51993303e-02, 2.80004182e-01,
4.05427058e-01, 2.14009347e-01, 3.99051249e-02, 1.51809635e-01,
8.09081900e+00, 6.68023804e+00, 5.29784122e+00, 5.67160924e+00,
5.93496227e+00, 8.37492511e+00, 6.67638890e+00, 4.15534146e+00,
1.02996749e+00, 3.56838401e+00, 2.59138009e+00, 2.81248790e+00,
2.34851585e+00, 2.75524166e+00, 3.08378139e+00, 2.96666935e+00,
3.03985353e+00, 3.06431010e+00, 2.35788341e+00, 3.27628910e+00,
3.36649771e+00, 2.96805503e+00, 3.32917200e+00, 1.68704987e+00,
1.54107353e+00, 1.57242053e+00, 1.74782160e+00, 8.46188075e-01,
8.16646613e-02, 7.94058502e-02, 8.27467516e-04, 2.47210927e-02,
9.58784409e-02, 1.18025764e-01, 3.29121479e-01, 1.53582345e-01,
2.09053372e-01, 1.67478606e-01, 1.38255244e-01, 2.60989359e-01,
1.39764497e-01, 1.40151347e-01, 5.50950458e-02, 3.13346899e-02,
1.34310492e-01, 2.59607171e-02, 1.20140067e-01, 2.94745341e-01,
6.92054431e-01, 1.55996606e-01]), surface_x=759587.9344606006, surface_y=3311661.864846832, x_points=array([759587.9344606 , 759587.9344606 , 759587.27126385, 759587.25110387,
759587.14384671, 759586.90140342, 759586.69872856, 759586.70697607,
759586.67468794, 759586.51852663, 759586.43237509, 759586.3658279 ,
759586.30865933, 759586.19064163, 759586.02340762, 759585.90439071,
759585.68456896, 759585.38136324, 759585.21476538, 759585.08752459,
759585.22736575, 759585.29981322, 759585.01791379, 759584.83875419,
759584.76875096, 759584.67835251, 759584.58475961, 759584.40919035,
759584.48227359, 759584.52239646, 759584.01838321, 759583.44130502,
759582.92466047, 759582.36642762, 759581.78733065, 759581.22328732,
759580.84625288, 759580.3978841 , 759579.82117412, 759579.34270204,
759578.87894355, 759578.42392781, 759577.98014809, 759577.57236517,
759577.17746828, 759576.82980699, 759576.63342616, 759576.56932604,
759576.60214568, 759576.72625098, 759576.93909277, 759576.88866017,
759576.81885493, 759576.90688553, 759577.02337706, 759577.28283845,
759577.15469638, 759577.14730817, 759577.59661448, 759578.03292036,
759578.68521334, 759581.22161098, 759586.02360369, 759592.86942965,
759601.37733639, 759612.45910442, 759625.36105102, 759640.18028782,
759655.3366563 , 759671.49004631, 759688.04636445, 759705.77344376,
759723.60567142, 759741.87373661, 759760.93464927, 759780.0829725 ,
759800.19053315, 759820.03608412, 759840.67111307, 759861.42355203,
759882.80253343, 759904.26669103, 759925.86331247, 759947.23433362,
759968.30005992, 759989.39966712, 760004.12794428, 760018.93982235,
760039.77842878, 760059.61605247, 760078.70125481, 760095.78407999,
760114.36110192, 760132.92846548, 760150.7958076 , 760169.17939146,
760187.54147056, 760204.8747981 , 760221.21535794, 760236.94835355,
760252.08342642, 760266.55095963, 760280.55658347, 760294.29579268,
760307.99697614, 760321.92830155, 760334.99760469, 760348.01029592,
760354.76280322, 760360.19547634]), y_points=array([3311661.86484683, 3311661.86484683, 3311661.24878415,
3311661.21944069, 3311661.08964549, 3311660.95817106,
3311660.92092015, 3311660.78371354, 3311660.57117851,
3311660.27611857, 3311660.21384569, 3311660.13832179,
3311659.59886925, 3311658.96182082, 3311658.32388916,
3311657.70192674, 3311657.1188912 , 3311656.58776023,
3311656.02863808, 3311655.43074019, 3311655.05469465,
3311654.76943716, 3311654.26786217, 3311653.76026365,
3311653.32715759, 3311652.85490447, 3311652.37235669,
3311651.8429922 , 3311651.36110864, 3311650.95556239,
3311650.5932407 , 3311650.339864 , 3311650.06669906,
3311649.70171798, 3311649.27279637, 3311648.8038419 ,
3311648.26573037, 3311648.22606883, 3311648.12609483,
3311647.37149678, 3311646.54694752, 3311645.70792198,
3311644.8398767 , 3311644.00660974, 3311643.27078717,
3311642.53052506, 3311641.85619832, 3311641.30685573,
3311640.81757807, 3311640.35802023, 3311639.95954258,
3311639.73413009, 3311639.56043741, 3311639.20716564,
3311638.77170149, 3311638.43442571, 3311638.46616955,
3311638.55097335, 3311638.3457934 , 3311638.23799242,
3311638.61795814, 3311640.70471308, 3311644.75760613,
3311650.49443777, 3311657.88069952, 3311667.55595319,
3311678.90437681, 3311691.94450126, 3311705.01191889,
3311718.74677073, 3311732.78727581, 3311747.7914497 ,
3311763.04547241, 3311778.81637118, 3311795.16690988,
3311811.63259591, 3311829.19071482, 3311846.6188331 ,
3311864.67697711, 3311882.76406144, 3311901.1811264 ,
3311919.50019834, 3311937.99793892, 3311956.93598039,
3311976.29342043, 3311995.6580847 , 3312008.9078354 ,
3312022.06536256, 3312041.7095322 , 3312062.37433193,
3312083.73870914, 3312103.62036075, 3312125.43284327,
3312147.65096509, 3312169.65631975, 3312192.02490221,
3312214.41109774, 3312237.21382663, 3312260.74231788,
3312285.04785793, 3312309.73153237, 3312334.46000232,
3312359.4534801 , 3312384.59550485, 3312409.41002521,
3312435.13788269, 3312460.63362103, 3312486.5008411 ,
3312499.82227577, 3312510.3953785 ]), zone_number=38, zone_letter='R', latitude_points=array([29.90829449, 29.90829449, 29.90828908, 29.90828882, 29.90828767,
29.90828654, 29.90828625, 29.90828501, 29.9082831 , 29.90828047,
29.90827993, 29.90827926, 29.90827441, 29.90826869, 29.90826298,
29.90825739, 29.90825219, 29.90824746, 29.90824246, 29.90823709,
29.90823367, 29.90823109, 29.90822662, 29.90822209, 29.9082182 ,
29.90821396, 29.90820963, 29.90820489, 29.90820053, 29.90819687,
29.90819371, 29.90819155, 29.90818919, 29.90818602, 29.90818228,
29.90817817, 29.9081734 , 29.90817313, 29.90817235, 29.90816565,
29.90815832, 29.90815085, 29.90814312, 29.90813569, 29.90812914,
29.90812254, 29.90811651, 29.90811157, 29.90810715, 29.90810298,
29.90809934, 29.90809732, 29.90809577, 29.90809257, 29.90808862,
29.90808552, 29.90808584, 29.9080866 , 29.90808466, 29.90808359,
29.90808688, 29.90810516, 29.90814068, 29.90819095, 29.90825574,
29.90834062, 29.9084402 , 29.90855463, 29.90866923, 29.90878963,
29.90891271, 29.90904422, 29.90917797, 29.90931628, 29.90945965,
29.90960404, 29.90975808, 29.90991099, 29.91006942, 29.91022809,
29.91038959, 29.9105502 , 29.91071239, 29.91087859, 29.91104864,
29.91121875, 29.91133508, 29.91145056, 29.91162324, 29.91180533,
29.91199389, 29.9121695 , 29.9123622 , 29.91255857, 29.91275316,
29.91295092, 29.91314884, 29.91335073, 29.91355937, 29.91377515,
29.91399446, 29.91421432, 29.91443666, 29.9146604 , 29.9148812 ,
29.91511018, 29.91533725, 29.91556768, 29.91568634, 29.9157805 ]), longitude_points=array([47.68852083, 47.68852083, 47.68851382, 47.6885136 , 47.68851246,
47.68850992, 47.68850782, 47.68850787, 47.68850748, 47.6885058 ,
47.68850489, 47.68850418, 47.68850346, 47.68850208, 47.6885002 ,
47.68849882, 47.6884964 , 47.68849314, 47.68849128, 47.68848981,
47.68849117, 47.68849185, 47.68848881, 47.68848684, 47.68848601,
47.68848496, 47.68848387, 47.68848193, 47.68848257, 47.68848288,
47.68847758, 47.68847155, 47.68846613, 47.68846027, 47.68845417,
47.68844822, 47.68844419, 47.68843954, 47.68843355, 47.68842842,
47.68842342, 47.68841851, 47.68841371, 47.68840928, 47.68840502,
47.68840124, 47.68839905, 47.68839825, 47.68839847, 47.68839965,
47.68840175, 47.68840118, 47.68840041, 47.68840124, 47.68840234,
47.68840494, 47.68840362, 47.68840356, 47.68840816, 47.68841265,
47.68841949, 47.68844624, 47.68849691, 47.68856914, 47.68865896,
47.68877598, 47.68891223, 47.68906873, 47.68922872, 47.6893992 ,
47.68957391, 47.68976098, 47.6899492 , 47.69014205, 47.69034324,
47.69054537, 47.69075769, 47.69096727, 47.69118517, 47.69140429,
47.69162998, 47.69185653, 47.69208449, 47.69231022, 47.6925329 ,
47.69275593, 47.69291155, 47.69306801, 47.69328841, 47.6934987 ,
47.69370137, 47.69388296, 47.69408049, 47.69427802, 47.69446825,
47.69466391, 47.69485935, 47.69504425, 47.69521906, 47.69538777,
47.69555038, 47.6957061 , 47.6958571 , 47.69600538, 47.69615319,
47.6963036 , 47.69644504, 47.69658599, 47.6966591 , 47.69671789]), isHorizontal=array(['Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Vertical', 'Vertical', 'Vertical', 'Vertical', 'Vertical',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal', 'Horizontal', 'Horizontal', 'Horizontal',
'Horizontal'], dtype='<U10'))
As you can see above, all the survey points have been calculated.
Deserialize Dict:
[17]:
# serialize
json_ds = well_obj.serialize()
json_ds
[17]:
'{"wellId": "well_C", "md": [0.0, 35.0, 774.81, 800.0, 900.0, 1000.0, 1100.0, 1200.0, 1300.0, 1400.0, 1500.0, 1600.0, 1700.0, 1800.0, 1900.0, 2000.0, 2100.0, 2200.0, 2300.0, 2400.0, 2500.0, 2600.0, 2700.0, 2800.0, 2900.0, 3000.0, 3100.0, 3200.0, 3300.0, 3400.0, 3500.0, 3600.0, 3700.0, 3800.0, 3900.0, 4000.0, 4100.0, 4200.0, 4300.0, 4400.0, 4500.0, 4600.0, 4700.0, 4800.0, 4900.0, 5000.0, 5100.0, 5200.0, 5300.0, 5400.0, 5500.0, 5600.0, 5700.0, 5800.0, 5900.0, 6000.0, 6100.0, 6200.0, 6300.0, 6400.0, 6450.67, 6532.0, 6625.0, 6720.0, 6813.0, 6909.0, 7002.0, 7098.0, 7191.0, 7286.0, 7379.0, 7475.0, 7569.0, 7663.0, 7758.0, 7851.0, 7947.0, 8040.0, 8135.0, 8229.0, 8324.0, 8418.0, 8512.0, 8606.0, 8700.0, 8794.0, 8859.0, 8924.0, 9018.0, 9112.0, 9206.0, 9292.0, 9386.0, 9481.0, 9574.0, 9669.0, 9764.0, 9858.0, 9952.0, 10047.0, 10142.0, 10236.0, 10330.0, 10424.0, 10517.0, 10613.0, 10707.0, 10802.0, 10851.0, 10890.0], "inc": [0.0, 0.0, 0.46, 0.13, 0.57, 0.49, 0.29, 0.6, 0.53, 0.83, 0.61, 0.87, 1.17, 1.27, 1.21, 1.18, 1.24, 1.07, 1.14, 1.16, 0.86, 0.95, 1.22, 0.82, 0.83, 0.98, 0.87, 1.25, 1.06, 1.07, 1.34, 1.03, 1.17, 1.34, 1.37, 1.39, 1.16, 1.61, 1.58, 1.78, 1.78, 1.81, 1.86, 1.63, 1.51, 1.57, 1.09, 0.99, 0.88, 0.91, 0.83, 0.77, 0.64, 0.91, 0.94, 0.66, 1.09, 0.91, 0.95, 0.8, 4.91, 10.35, 15.28, 20.67, 26.19, 34.23, 40.44, 44.43, 45.39, 48.78, 51.19, 53.89, 56.1, 58.69, 61.62, 64.38, 67.3, 70.15, 72.39, 75.47, 78.67, 81.46, 84.59, 86.2, 87.65, 89.13, 90.27, 89.72, 89.9, 90.02, 90.05, 90.03, 89.94, 90.06, 90.37, 90.24, 90.06, 89.97, 89.88, 90.15, 90.03, 89.91, 89.97, 90.0, 89.88, 89.88, 90.0, 89.72, 90.06, 90.0], "azim": [227.11, 227.11, 227.11, 163.86, 230.43, 254.47, 268.25, 147.67, 236.56, 190.05, 342.93, 184.69, 187.06, 193.66, 195.77, 185.77, 214.81, 203.82, 189.81, 194.18, 109.65, 214.46, 205.35, 190.63, 187.75, 193.45, 188.19, 205.4, 130.09, 218.09, 247.16, 245.17, 239.46, 234.52, 232.45, 228.1, 199.28, 305.98, 213.22, 211.63, 207.08, 209.84, 204.39, 208.0, 208.46, 201.98, 187.95, 185.23, 165.95, 163.86, 138.75, 253.13, 132.16, 189.07, 141.79, 143.34, 306.53, 111.26, 117.69, 87.41, 55.44, 48.23, 50.93, 49.37, 48.77, 48.96, 48.41, 48.88, 49.58, 49.67, 49.73, 49.78, 49.14, 49.25, 49.5, 49.12, 48.63, 48.79, 48.83, 49.02, 49.49, 49.55, 49.29, 47.62, 47.22, 47.69, 48.36, 48.41, 44.97, 42.69, 40.86, 40.48, 40.36, 39.41, 38.74, 40.09, 38.63, 35.85, 33.71, 32.12, 30.91, 29.75, 28.78, 28.53, 29.28, 27.59, 26.69, 26.72, 27.04, 27.35], "tvd": [0.0, 34.9999999999999, 774.8020523575979, 799.9916838982401, 899.9895706619063, 999.9852843864701, 1099.9829170820146, 1199.9797718735474, 1299.9749056242865, 1399.9677450917852, 1499.9598074391574, 1599.951358560927, 1699.9353985310372, 1799.9127169630583, 1899.889294307163, 1999.8675439283443, 2099.845239700057, 2199.824885701308, 2299.806282545446, 2399.786139863118, 2499.770513641399, 2599.7580217806603, 2699.7399992785035, 2799.7239516033837, 2899.7135851343646, 2999.701082311137, 3099.6880353482165, 3199.6707375309666, 3299.6503914295877, 3399.6331165071, 3499.610904896245, 3599.5893961454594, 3699.5709423951766, 3799.5469175712324, 3899.5189534454853, 3999.489948634858, 4099.465126923902, 4199.4355637827375, 4299.396829968807, 4399.35379463453, 4499.305540982791, 4599.256469555635, 4699.205184887019, 4799.158743060025, 4899.121184533607, 4999.085060495655, 5099.05782961302, 5199.041343661597, 5299.028013859704, 5399.015812645296, 5499.004276998297, 5598.994530251943, 5698.98694779626, 5798.9777010953785, 5898.964667638501, 5998.9548205379915, 6098.942872857037, 6198.927635252819, 6298.914460300661, 6398.9027722133005, 6449.498920678799, 6530.078406955509, 6620.7338571887985, 6711.063657145683, 6796.362470827802, 6879.256358828799, 6953.164725618479, 7024.002532987769, 7089.865880174081, 7154.543119676297, 7214.336581582896, 7272.719061079499, 7326.638576597015, 7377.28559393612, 7424.557660836965, 7466.774650295593, 7506.061815793666, 7539.80284575592, 7570.306200996387, 7596.323302940914, 7617.577725120327, 7633.793966038078, 7645.207495361553, 7652.754047905717, 7657.7963045183415, 7660.437202287923, 7660.7774906349405, 7660.783119177495, 7661.09477092539, 7661.160332020118, 7661.102847387391, 7661.042750144869, 7661.067296013801, 7661.06723205933, 7660.71819198431, 7660.212421103769, 7659.9636479664405, 7659.938975524531, 7660.061957987962, 7660.037023111568, 7659.887733518575, 7659.93688853576, 7660.035261841736, 7660.059807712877, 7660.157134486219, 7660.358131730846, 7660.456505021519, 7660.688569495339, 7660.782609598131, 7660.7621629839505], "e_w_deviation": [0.0, -1.484000657881275e-17, -2.175842355175559, -2.2419840214828732, -2.593877605762808, -3.389295211600157, -4.0542389625514135, -4.027180204159597, -4.13311241136453, -4.645452673436392, -4.92810207452572, -5.146432756144027, -5.3339936813724895, -5.721190851580817, -6.269858859107042, -6.660334283164571, -7.3815342656473435, -8.37630368630489, -8.922884580145645, -9.340341250202624, -8.881544780257427, -8.643856233265076, -9.568723135321147, -10.156517109805641, -10.386186489246885, -10.682769333247977, -10.989832648807775, -11.56584727461761, -11.326072883057673, -11.194436173167277, -12.848022933081339, -14.74132409432717, -16.436352123638066, -18.267824737470026, -20.16774917889891, -22.018285039135044, -23.25527466581436, -24.726300840835037, -26.61839395085467, -28.188184254357466, -29.70970162039467, -31.202535397595472, -32.6585055963508, -33.99637609819523, -35.29196955641501, -36.43259059281338, -37.076884648839126, -37.28718688470833, -37.179510885615926, -36.772341260215114, -36.07404142749863, -36.23950271973721, -36.46852254598554, -36.17970824196029, -35.79751817472034, -34.946266913548605, -35.366680506690855, -35.39092003219164, -33.91681798193058, -32.48536824485758, -30.345299422455874, -22.023784850465717, -6.26921560023823, 16.190843339757308, 44.10392321003876, 80.4614298575519, 122.79065099746501, 171.4101943000012, 221.13581267417885, 274.1324990599122, 328.4511281163804, 386.6108371411615, 445.11552106990206, 505.050118137853, 567.5859208385572, 630.4085035962628, 696.3781907690641, 761.4882661350741, 829.1884923449869, 897.273922021971, 967.4149370948395, 1037.8354016688197, 1108.6904588935624, 1178.8053576711623, 1247.9186329320553, 1317.143065996658, 1365.4641853124037, 1414.059585780118, 1482.4277171087615, 1547.5117843566725, 1610.1272775774444, 1666.1732919750543, 1727.121526626948, 1788.0380737677126, 1846.657962591831, 1906.9715579444687, 1967.2145995930707, 2024.0824721238525, 2077.6932327252953, 2129.310672394236, 2178.966423288364, 2226.4320834376203, 2272.382292878736, 2317.4584385834237, 2362.4098278862716, 2408.116276072377, 2450.9945672173526, 2493.6871237531554, 2515.841019107563, 2533.664749805278], "n_s_deviation": [0.0, -1.3785312281415713e-17, -2.0212030353173684, -2.117474233940387, -2.5433114844573717, -2.9746580295078346, -3.096872330184516, -3.547025241268128, -4.244318643030875, -5.212363072305503, -5.416670419759379, -5.6644522515964795, -7.4343096514447415, -9.524363563889594, -11.617315201593373, -13.657874309319288, -15.570720570759654, -17.313276242698638, -19.147666498237268, -21.1092737561569, -22.343018956190242, -23.278903111169665, -24.92449035064133, -26.58983984591266, -28.010791491809098, -29.560178344151375, -31.14334036107004, -32.880100514039235, -34.46108331932539, -35.79161560995158, -36.98033508016032, -37.81162348161602, -38.70783391216995, -39.90527839354168, -41.31250151308184, -42.851066051697146, -44.616523833118926, -44.74664698463293, -45.07464567742085, -47.55036106024729, -50.255575155570206, -53.0082836249512, -55.85620121029757, -58.59001671264978, -61.00413274169478, -63.432814219688424, -65.64517228195949, -67.44747737684362, -69.05271904531232, -70.56045471772394, -71.8677961030382, -72.60733839941273, -73.17719626309531, -74.33622436740495, -75.76491253054486, -76.87146038506376, -76.76731390609072, -76.48908622388853, -77.16224878848631, -77.51592653796689, -76.26931984432156, -69.42301100110615, -56.12611778437354, -37.30449166745683, -13.071349447776281, 18.671608779555633, 55.903969746441945, 98.68653026453202, 141.5586353467743, 186.6204852148685, 232.68513445656907, 281.9114267345113, 331.9574329881619, 383.69922686653683, 437.3427265322022, 491.3640061689003, 548.9693831616727, 606.1482488956053, 665.3941282074068, 724.7349560515827, 785.1583975473234, 845.2603395856305, 905.9484648652372, 968.0811468556537, 1031.5898084053179, 1095.1221714720284, 1138.5924821915255, 1181.7602222204512, 1246.2095976634116, 1314.0074970566761, 1384.100598108905, 1449.3291138949253, 1520.8923767738684, 1593.786477219505, 1665.9825226964995, 1739.3702604404925, 1812.8157838142843, 1887.627886485367, 1964.8210992507668, 2044.5636846900316, 2125.546868561508, 2206.677019325788, 2288.6766183469813, 2371.1635761844777, 2452.576044537638, 2536.9850257909825, 2620.632461288653, 2705.4986688433305, 2749.2041631671486, 2783.892820441016], "dls": [0.0, 0.0, 0.062178175697026876, 1.312323104221892, 0.43922131729609876, 0.08042287727355951, 0.20007154008744296, 0.30541848464608146, 0.07544251812726605, 0.2976066556324211, 0.23670095082420844, 0.24213627593834536, 0.2999850410059419, 0.09982823459047929, 0.06001822909970222, 0.03037855739338735, 0.056789950199735574, 0.17042476568489642, 0.0693668431679535, 0.01993292540609921, 0.3157502687404893, 0.0720972968028324, 0.26974508589212887, 0.40057331440554544, 0.009985005553010146, 0.14992993001118987, 0.11006274453029032, 0.37915058010860575, 0.2072590379355382, 0.009102498691501362, 0.26684825484593366, 0.3100147696535474, 0.1398957617883856, 0.16989850673084672, 0.029979119030003634, 0.019904290966461985, 0.23348528080380748, 0.40804615992287036, 0.07652363332976642, 0.19998126332911942, 0.00017422010087074383, 0.029934816672579877, 0.04973451056650544, 0.23010514458114228, 0.1200014784499837, 0.059735768555311125, 0.4808912031722217, 0.10002129508581828, 0.11085278275925033, 0.029990726732150582, 0.08124578232425676, 0.07575783435644577, 0.14302641690198467, 0.26538522507724854, 0.02519933025313245, 0.28000418182841885, 0.4054270579062658, 0.21400934683013106, 0.039905124947546486, 0.15180963459746707, 8.09081900129934, 6.6802380356223, 5.297841217404203, 5.671609243355286, 5.934962274534154, 8.374925113240641, 6.676388904579787, 4.155341460623003, 1.0299674900525506, 3.5683840146139207, 2.591380087028445, 2.8124879045508773, 2.3485158500356245, 2.7552416559590474, 3.083781392084175, 2.9666693510617885, 3.0398535258486246, 3.0643100952872393, 2.357883414036999, 3.276289102066852, 3.366497713956813, 2.968055033124839, 3.3291719989157795, 1.6870498749295495, 1.541073533810662, 1.5724205308300976, 1.7478215994323207, 0.8461880746380327, 0.08166466134158418, 0.07940585015175593, 0.0008274675158041158, 0.024721092669823027, 0.09587844093486547, 0.11802576377694084, 0.32912147949062376, 0.15358234453355382, 0.20905337189170117, 0.16747860550934596, 0.13825524436433095, 0.26098935876969753, 0.1397644970882708, 0.14015134747360147, 0.0550950458378559, 0.031334689863031874, 0.13431049244001467, 0.025960717101329234, 0.12014006714273541, 0.29474534107044165, 0.6920544308284147, 0.15599660612029712], "surface_latitude": 29.90829443997491, "surface_longitude": 47.68852083021084, "longitude_points": [47.688520831501584, 47.688520831501584, 47.688513819911336, 47.68851360419968, 47.68851246292549, 47.688509922425304, 47.688507816251104, 47.68850786834965, 47.68850748276132, 47.68850579541948, 47.68850488889173, 47.68850418200681, 47.68850345976884, 47.68850208425861, 47.68850019927591, 47.6884988170818, 47.688496401255236, 47.688493135200524, 47.68849127589454, 47.688489814435975, 47.6884911703171, 47.68849185084708, 47.68848881241754, 47.68848683561406, 47.68848600633745, 47.688484956540215, 47.68848387119491, 47.68848192626939, 47.68848256574146, 47.68848288265531, 47.688477579675016, 47.688471547060516, 47.688466134980224, 47.688460270327404, 47.68845417429598, 47.68844822433165, 47.68844419265932, 47.68843954362338, 47.68843355198496, 47.6884284182561, 47.688423419827906, 47.688418508357735, 47.68841370612089, 47.6884092847814, 47.68840502038599, 47.68840124367845, 47.68839904829562, 47.688398251945436, 47.68839847300854, 47.68839964583533, 47.68840175164598, 47.68840117519401, 47.68840041081649, 47.688401236114935, 47.688402335996216, 47.688404939022064, 47.68840362078311, 47.68840356487949, 47.68840816429657, 47.6884126527849, 47.68841949433224, 47.68844624484414, 47.68849691458778, 47.688569140596954, 47.68865896444639, 47.68877597569467, 47.68891222662516, 47.689068726695666, 47.689228722167776, 47.68939919634203, 47.68957391426741, 47.68976098056631, 47.68994919593216, 47.690142046907624, 47.6903432429029, 47.69054537186555, 47.69075769200728, 47.69096727012203, 47.691185170809234, 47.691404294102114, 47.691629981304615, 47.69185652681985, 47.692084487080734, 47.692310220485574, 47.69253289733271, 47.69275592727063, 47.692911545639134, 47.69306800705455, 47.693288405880466, 47.69349869537966, 47.69370136965815, 47.693882964679226, 47.69408049072123, 47.694278016092035, 47.69446824691487, 47.69466390866511, 47.69485935294891, 47.69504425385438, 47.69521905882091, 47.695387766321765, 47.69555037920689, 47.69570609607321, 47.69585709823844, 47.696005380354435, 47.696153190080246, 47.69630360397242, 47.696445041941594, 47.696585985057574, 47.69665909843736, 47.69671788618887], "latitude_points": [29.908294493985753, 29.908294493985753, 29.908289080306787, 29.908288820037562, 29.90828767260317, 29.908286538564674, 29.908286245535, 29.908285006908837, 29.908283097770482, 29.90828047083531, 29.908279927643903, 29.908279260859743, 29.90827440988825, 29.90826869195605, 29.908262976449773, 29.90825739472529, 29.908252185194232, 29.908247461170397, 29.90824245597889, 29.908237092926104, 29.90823367344771, 29.90823108662694, 29.908226624546064, 29.90822208647922, 29.908218196905043, 29.908213958734137, 29.908209628433415, 29.90820489339357, 29.90820053390071, 29.908196869527742, 29.908193709663326, 29.908191547333807, 29.908189193862192, 29.908186021467223, 29.908182277066768, 29.90817816860179, 29.908173397230893, 29.90817313432603, 29.908172354806403, 29.90816565326943, 29.908158318032953, 29.908150850450646, 29.908143118890433, 29.908135693250845, 29.908129143329806, 29.908122543417527, 29.908116505967776, 29.908111567301635, 29.9081071496509, 29.90810298065067, 29.908099343545203, 29.908097322148585, 29.908095771082802, 29.90809256784058, 29.908088617646207, 29.9080855224217, 29.90808583563081, 29.908086601676597, 29.90808465719632, 29.90808359330845, 29.908086880936587, 29.90810515720459, 29.90814067954787, 29.908190950781172, 29.908255740359994, 29.908340621272984, 29.908440201054653, 29.908554626186906, 29.90866922597247, 29.908789631783172, 29.908912707654125, 29.90904422326989, 29.909177968706487, 29.909316281299017, 29.909459651416338, 29.909604040747578, 29.909758075090956, 29.909910992481457, 29.910069422205964, 29.91022808760332, 29.910389594872917, 29.910550200323797, 29.910712387988628, 29.91087859209313, 29.91104864106646, 29.91121874757363, 29.911335076508863, 29.911450556188566, 29.91162323652526, 29.911805328832266, 29.91199388638588, 29.912169500757, 29.91236220465969, 29.912558566980355, 29.9127531590173, 29.91295091589507, 29.913148835755887, 29.913350727900745, 29.913559372370162, 29.913775150011364, 29.913994462711045, 29.914214320277512, 29.91443666435734, 29.91466040372188, 29.914881198568317, 29.915110177933432, 29.915337247116263, 29.91556767688253, 29.91568633568558, 29.915780498555556], "zone_number": 38, "zone_letter": "R", "x_points": [759587.9344606006, 759587.9344606006, 759587.2712638507, 759587.2511038708, 759587.1438467064, 759586.9014034201, 759586.6987285648, 759586.7069760744, 759586.6746879376, 759586.5185266257, 759586.4323750883, 759586.3658278965, 759586.3086593265, 759586.190641629, 759586.0234076204, 759585.9043907111, 759585.6845689564, 759585.381363237, 759585.2147653806, 759585.0875245875, 759585.2273657516, 759585.2998132207, 759585.0179137889, 759584.8387541856, 759584.7687509587, 759584.6783525079, 759584.5847596092, 759584.4091903513, 759584.4822735859, 759584.522396455, 759584.0183832106, 759583.4413050166, 759582.9246604734, 759582.3664276206, 759581.7873306508, 759581.2232873207, 759580.8462528825, 759580.3978841043, 759579.8211741244, 759579.3427020399, 759578.8789435467, 759578.4239278114, 759577.9801480948, 759577.5723651659, 759577.1774682798, 759576.8298069879, 759576.6334261596, 759576.5693260381, 759576.6021456827, 759576.7262509845, 759576.9390927735, 759576.8886601717, 759576.8188549286, 759576.9068855285, 759577.023377061, 759577.2828384454, 759577.1546963821, 759577.1473081748, 759577.5966144797, 759578.0329203595, 759578.6852133366, 759581.2216109781, 759586.0236036857, 759592.8694296506, 759601.377336395, 759612.4591044212, 759625.3610510246, 759640.1802878232, 759655.3366563037, 759671.4900463141, 759688.0463644505, 759705.7734437613, 759723.6056714227, 759741.873736609, 759760.9346492722, 759780.0829724967, 759800.190533147, 759820.0360841185, 759840.6711130673, 759861.4235520329, 759882.8025334271, 759904.2666910293, 759925.8633124713, 759947.2343336188, 759968.3000599183, 759989.3996671163, 760004.1279442838, 760018.9398223464, 760039.7784287754, 760059.6160524725, 760078.7012548062, 760095.7840799947, 760114.3611019165, 760132.928465485, 760150.7958075986, 760169.1793914621, 760187.5414705565, 760204.8747981039, 760221.2153579353, 760236.9483535463, 760252.083426419, 760266.5509596324, 760280.5565834701, 760294.2957926808, 760307.9969761403, 760321.9283015474, 760334.9976046884, 760348.0102959205, 760354.7628032246, 760360.1954763413], "y_points": [3311661.864846832, 3311661.864846832, 3311661.2487841467, 3311661.2194406856, 3311661.0896454914, 3311660.9581710645, 3311660.9209201457, 3311660.7837135387, 3311660.57117851, 3311660.2761185677, 3311660.2138456884, 3311660.1383217857, 3311659.59886925, 3311658.961820818, 3311658.3238891587, 3311657.7019267427, 3311657.1188912024, 3311656.5877602333, 3311656.0286380835, 3311655.430740191, 3311655.0546946544, 3311654.7694371636, 3311654.2678621733, 3311653.760263647, 3311653.3271575854, 3311652.854904473, 3311652.37235669, 3311651.8429921954, 3311651.3611086365, 3311650.955562394, 3311650.5932406997, 3311650.339863995, 3311650.0666990555, 3311649.701717978, 3311649.272796371, 3311648.8038418996, 3311648.265730368, 3311648.226068831, 3311648.1260948298, 3311647.371496781, 3311646.546947525, 3311645.707921983, 3311644.8398767035, 3311644.006609738, 3311643.2707871725, 3311642.530525058, 3311641.8561983206, 3311641.3068557275, 3311640.817578067, 3311640.3580202344, 3311639.95954258, 3311639.734130088, 3311639.560437411, 3311639.207165645, 3311638.771701493, 3311638.434425707, 3311638.4661695533, 3311638.550973351, 3311638.3457934014, 3311638.237992423, 3311638.6179581434, 3311640.704713079, 3311644.7576061315, 3311650.494437772, 3311657.8806995205, 3311667.5559531883, 3311678.904376811, 3311691.9445012566, 3311705.0119188856, 3311718.7467707256, 3311732.7872758145, 3311747.791449701, 3311763.045472407, 3311778.816371181, 3311795.166909879, 3311811.6325959126, 3311829.19071482, 3311846.6188330953, 3311864.6769771096, 3311882.7640614365, 3311901.1811264046, 3311919.5001983377, 3311937.997938923, 3311956.9359803936, 3311976.293420434, 3311995.6580846966, 3312008.907835404, 3312022.0653625648, 3312041.7095322, 3312062.374331935, 3312083.738709136, 3312103.6203607474, 3312125.4328432726, 3312147.6509650885, 3312169.65631975, 3312192.0249022143, 3312214.411097739, 3312237.213826633, 3312260.7423178838, 3312285.0478579258, 3312309.7315323697, 3312334.4600023227, 3312359.4534801045, 3312384.595504853, 3312409.4100252073, 3312435.137882693, 3312460.633621033, 3312486.5008410956, 3312499.8222757652, 3312510.3953785026], "surface_x": 759587.9344606006, "surface_y": 3311661.864846832, "isHorizontal": ["Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Vertical", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal", "Horizontal"]}'
Now you have done it, you can check out your calculated points in a dataframe for easy viewing.
[18]:
json_ds_obj = json.loads(json_ds)
dfXY = pd.DataFrame(json_ds_obj)
dfXY.head()
[18]:
wellId | md | inc | azim | tvd | e_w_deviation | n_s_deviation | dls | surface_latitude | surface_longitude | longitude_points | latitude_points | zone_number | zone_letter | x_points | y_points | surface_x | surface_y | isHorizontal | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | well_C | 0.00 | 0.00 | 227.11 | 0.000000 | 0.000000e+00 | 0.000000e+00 | 0.000000 | 29.908294 | 47.688521 | 47.688521 | 29.908294 | 38 | R | 759587.934461 | 3.311662e+06 | 759587.934461 | 3.311662e+06 | Vertical |
1 | well_C | 35.00 | 0.00 | 227.11 | 35.000000 | -1.484001e-17 | -1.378531e-17 | 0.000000 | 29.908294 | 47.688521 | 47.688521 | 29.908294 | 38 | R | 759587.934461 | 3.311662e+06 | 759587.934461 | 3.311662e+06 | Vertical |
2 | well_C | 774.81 | 0.46 | 227.11 | 774.802052 | -2.175842e+00 | -2.021203e+00 | 0.062178 | 29.908294 | 47.688521 | 47.688514 | 29.908289 | 38 | R | 759587.271264 | 3.311661e+06 | 759587.934461 | 3.311662e+06 | Vertical |
3 | well_C | 800.00 | 0.13 | 163.86 | 799.991684 | -2.241984e+00 | -2.117474e+00 | 1.312323 | 29.908294 | 47.688521 | 47.688514 | 29.908289 | 38 | R | 759587.251104 | 3.311661e+06 | 759587.934461 | 3.311662e+06 | Vertical |
4 | well_C | 900.00 | 0.57 | 230.43 | 899.989571 | -2.593878e+00 | -2.543311e+00 | 0.439221 | 29.908294 | 47.688521 | 47.688512 | 29.908288 | 38 | R | 759587.143847 | 3.311661e+06 | 759587.934461 | 3.311662e+06 | Vertical |
[19]:
dfXY.plot(kind="scatter", x="longitude_points", y="latitude_points", alpha=0.4)
plt.show()
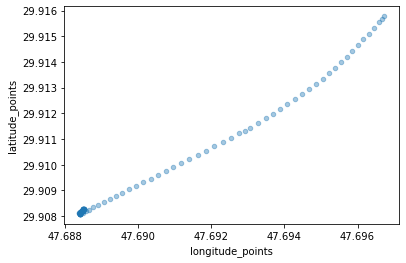
[20]:
fig = pyplot.figure()
ax = Axes3D(fig)
ax.scatter(dfXY['longitude_points'].values, dfXY['latitude_points'].values, dfXY['tvd'].values*-1)
pyplot.show()
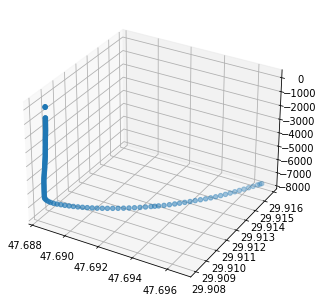
[19]:
# Finished